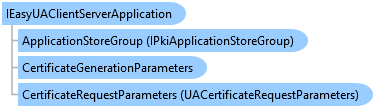
'Declaration
<ComVisibleAttribute(False)> <ExceptionContractAnnotationAttribute(True)> <CLSCompliantAttribute(True)> Public Interface IEasyUAClientServerApplication Inherits OpcLabs.BaseLib.ComponentModel.IEventLocking, System.IDisposable, System.IServiceProvider
'Usage
Dim instance As IEasyUAClientServerApplication
[ComVisible(false)] [ExceptionContractAnnotation(true)] [CLSCompliant(true)] public interface IEasyUAClientServerApplication : OpcLabs.BaseLib.ComponentModel.IEventLocking, System.IDisposable, System.IServiceProvider
[ComVisible(false)] [ExceptionContractAnnotation(true)] [CLSCompliant(true)] public interface class IEasyUAClientServerApplication : public OpcLabs.BaseLib.ComponentModel.IEventLocking, System.IDisposable, System.IServiceProvider
This service has high-level properties and methods for tasks related to maintaining application registrations in the GDS servers, obtaining own certificate from GDS, refreshing application trust lists from GDS, etc.
You can obtain this interface by calling System.IServiceProvider.GetService(System.Type) on the OpcLabs.EasyOpc.UA.IEasyUAClient with the type of IEasyUAClientServerApplication as an argument. In COM, use the OpcLabs.EasyOpc.UA.ComTypes._EasyUAClient.GetServiceByName method instead.
// Shows how to obtain a new application certificate pack from the certificate manager (GDS), and store it for subsequent // usage. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in C# on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-CSharp . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. using System; using System.Collections.Generic; using OpcLabs.BaseLib.Security.Cryptography.PkiCertificates; using OpcLabs.EasyOpc.UA; using OpcLabs.EasyOpc.UA.AddressSpace; using OpcLabs.EasyOpc.UA.Application; using OpcLabs.EasyOpc.UA.Application.Extensions; using OpcLabs.EasyOpc.UA.Extensions; using OpcLabs.EasyOpc.UA.OperationModel; namespace UADocExamples.Application._IEasyUAClientServerApplication { partial class ObtainNewCertificatePack { public static void Main1() { // Define which GDS we will work with. UAEndpointDescriptor gdsEndpointDescriptor = ((UAEndpointDescriptor)"opc.tcp://opcua.demo-this.com:58810/GlobalDiscoveryServer") .WithUserNameIdentity("appadmin", "demo"); // Obtain the application interface. EasyUAApplication application = EasyUAApplication.Instance; // Display which application we are about to work with. Console.WriteLine("Application URI string: {0}", application.GetApplicationElement().ApplicationUriString); // Obtain a new application certificate pack from the certificate manager (GDS), and store it for subsequent // usage. UANodeIdPkiCertificateDictionary certificateDictionary; try { certificateDictionary = application.ObtainNewCertificatePack(gdsEndpointDescriptor); } catch (UAException uaException) { Console.WriteLine("*** Failure: {0}", uaException.GetBaseException().Message); return; } // Display results foreach (KeyValuePair<UANodeId, IPkiCertificate> pair in certificateDictionary) { Console.WriteLine(); Console.WriteLine($"Certificate type Id: {pair.Key}"); Console.WriteLine($"Certificate: {pair.Value}"); } } } }
' Shows how to obtain a new application certificate pack from the certificate manager (GDS), and store it for subsequent ' usage. ' ' Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . ' OPC client and subscriber examples in VB.NET on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBNET . ' Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own ' a commercial license in order to use Online Forums, and we reply to every post. Imports OpcLabs.BaseLib.Security.Cryptography.PkiCertificates Imports OpcLabs.EasyOpc.UA Imports OpcLabs.EasyOpc.UA.AddressSpace Imports OpcLabs.EasyOpc.UA.Application Imports OpcLabs.EasyOpc.UA.Application.Extensions Imports OpcLabs.EasyOpc.UA.Extensions Imports OpcLabs.EasyOpc.UA.OperationModel Namespace Application._IEasyUAClientServerApplication Partial Friend Class ObtainNewCertificatePack Public Shared Sub Main1() ' Define which GDS we will work with. Dim gdsEndpointDescriptor As UAEndpointDescriptor = New UAEndpointDescriptor("opc.tcp://opcua.demo-this.com:58810/GlobalDiscoveryServer") _ .WithUserNameIdentity("appadmin", "demo") ' Obtain the application interface. Dim application As EasyUAApplication = EasyUAApplication.Instance ' Display which application we are about to work with. Console.WriteLine("Application URI string: {0}", application.GetApplicationElement().ApplicationUriString) ' Obtain a new application certificate pack from the certificate manager (GDS), and store it for subsequent ' usage. Dim certificateDictionary As UANodeIdPkiCertificateDictionary Try certificateDictionary = application.ObtainNewCertificatePack(gdsEndpointDescriptor) Catch uaException As UAException Console.WriteLine("*** Failure: {0}", uaException.GetBaseException.Message) Exit Sub End Try ' Display results For Each pair As KeyValuePair(Of UANodeId, IPkiCertificate) In certificateDictionary Console.WriteLine() Console.WriteLine($"Certificate type Id: {pair.Key}") Console.WriteLine($"Certificate: {pair.Value}") Next End Sub End Class End Namespace
// Shows how to obtain a new application certificate pack from the certificate manager (GDS), // and store it for subsequent usage. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in Object Pascal (Delphi) on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-OP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. class procedure ObtainNewCertificatePack.Main; var Application: TEasyUAApplication; ApplicationElement: _UAApplicationElement; CertificateDictionary: _UANodeIdPkiCertificateReadOnlyDictionary; GdsEndpointDescriptor: _UAEndpointDescriptor; Count: Cardinal; Element: OleVariant; Enumerator: IEnumVARIANT; CertificateTypeId: _UANodeId; Certificate: _PkiCertificate; S: string; begin // Define which GDS we will work with. GdsEndpointDescriptor := CoUAEndpointDescriptor.Create; GdsEndpointDescriptor.UrlString := 'opc.tcp://opcua.demo-this.com:58810/GlobalDiscoveryServer'; GdsEndpointDescriptor.UserIdentity.UserNameTokenInfo.UserName := 'appadmin'; GdsEndpointDescriptor.UserIdentity.UserNameTokenInfo.Password := 'demo'; // Obtain the application interface. Application := TEasyUAApplication.Create(nil); // Display which application we are about to work with. ApplicationElement := Application.GetApplicationElement; WriteLn('Application URI string: ', Application.GetApplicationElement.ApplicationUriString); // Obtain a new application certificate pack from the certificate manager (GDS), and store it for subsequent usage. try CertificateDictionary := Application.ObtainNewCertificatePack(GdsEndpointDescriptor); except on E: EOleException do begin WriteLn(Format('*** Failure: %s', [E.GetBaseException.Message])); Exit; end; end; // Display results Enumerator := CertificateDictionary.GetEnumerator; while (Enumerator.Next(1, Element, Count) = S_OK) do begin WriteLn; CertificateTypeId := IUnknown(Element.Key) as _UANodeId; WriteLn('Certificate type Id: ', CertificateTypeId.ToString); Certificate := IUnknown(Element.Value) as _PkiCertificate; if Certificate = nil then S := '' else S := (Certificate as _PkiCertificate).ToString; WriteLn('Certificate: ', S); end; end;
// Shows how to obtain a new application certificate pack from the certificate manager (GDS), // and store it for subsequent usage. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in PHP on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-PHP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. // Define which GDS we will work with. $GdsEndpointDescriptor = new COM("OpcLabs.EasyOpc.UA.UAEndpointDescriptor"); $GdsEndpointDescriptor->UrlString = "opc.tcp://opcua.demo-this.com:58810/GlobalDiscoveryServer"; $GdsEndpointDescriptor->UserIdentity->UserNameTokenInfo->UserName = "appadmin"; $GdsEndpointDescriptor->UserIdentity->UserNameTokenInfo->Password = "demo"; // Obtain the application interface. $Application = new COM("OpcLabs.EasyOpc.UA.Application.EasyUAApplication"); // Display which application we are about to work with. $ApplicationElement = $Application->GetApplicationElement; printf("Application URI string: %s\n", $Application->GetApplicationElement->ApplicationUriString); // Obtain a new application certificate pack from the certificate manager (GDS), and store it for subsequent usage. try { $CertificateDictionary = $Application->ObtainNewCertificatePack($GdsEndpointDescriptor); } catch (com_exception $e) { printf("*** Failure: %s\n", $e->getMessage()); exit(); } // Display results foreach ($CertificateDictionary as $Pair) { printf("\n"); printf("Certificate type Id: %s\n", $Pair->Key); printf("Certificate: %s\n", $Pair->Value); }
REM Shows how to obtain a new application certificate pack from the certificate manager (GDS), REM and store it for subsequent usage. REM REM Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . REM OPC client and subscriber examples in Visual Basic on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VB . REM Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own REM a commercial license in order to use Online Forums, and we reply to every post. Private Sub IEasyUAClientServerApplication_ObtainNewCertificatePack_Main_Command_Click() OutputText = "" ' Define which GDS we will work with. Dim gdsEndpointDescriptor As New UAEndpointDescriptor gdsEndpointDescriptor.UrlString = "opc.tcp://opcua.demo-this.com:58810/GlobalDiscoveryServer" gdsEndpointDescriptor.UserIdentity.UserNameTokenInfo.UserName = "appadmin" gdsEndpointDescriptor.UserIdentity.UserNameTokenInfo.Password = "demo" ' Obtain the application interface Dim Application As New EasyUAApplication ' Display which application we are about to work with. OutputText = OutputText & "Application URI string: " & Application.GetApplicationElement.applicationUriString & vbCrLf ' Obtain a new application certificate pack from the certificate manager (GDS), and store it for subsequent usage. On Error Resume Next Dim certificateDictionary As UANodeIdPkiCertificateDictionary Set certificateDictionary = Application.ObtainNewCertificatePack(gdsEndpointDescriptor) If Err.Number <> 0 Then OutputText = OutputText & "*** Failure: " & Err.Source & ": " & Err.Description & vbCrLf Exit Sub End If On Error GoTo 0 ' Display results Dim Pair : For Each Pair In certificateDictionary OutputText = OutputText & vbCrLf OutputText = OutputText & "Certificate type Id: " & Pair.Key & vbCrLf OutputText = OutputText & "Certificate: " & Pair.Value & vbCrLf Next End Sub
Rem Shows how to obtain a new application certificate pack from the certificate manager (GDS), and store it for subsequent Rem usage. Rem Rem Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . Rem OPC client and subscriber examples in VBScript on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBScript . Rem Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own Rem a commercial license in order to use Online Forums, and we reply to every post. Option Explicit ' Define which GDS we will work with. Dim GdsEndpointDescriptor: Set GdsEndpointDescriptor = CreateObject("OpcLabs.EasyOpc.UA.UAEndpointDescriptor") GdsEndpointDescriptor.UrlString = "opc.tcp://opcua.demo-this.com:58810/GlobalDiscoveryServer" GdsEndpointDescriptor.UserIdentity.UserNameTokenInfo.UserName = "appadmin" GdsEndpointDescriptor.UserIdentity.UserNameTokenInfo.Password = "demo" ' Obtain the application interface. Dim Application: Set Application = CreateObject("OpcLabs.EasyOpc.UA.Application.EasyUAApplication") ' Display which application we are about to work with. Dim ApplicationElement: Set ApplicationElement = Application.GetApplicationElement WScript.Echo "Application URI string: " & Application.GetApplicationElement.ApplicationUriString Rem Obtain a new application certificate pack from the certificate manager (GDS), and store it for subsequent usage. On Error Resume Next Dim CertificateDictionary: Set CertificateDictionary = Application.ObtainNewCertificatePack(GdsEndpointDescriptor) If Err.Number <> 0 Then WScript.Echo "*** Failure: " & Err.Source & ": " & Err.Description WScript.Quit End If On Error Goto 0 ' Display results Dim Pair: For Each Pair In CertificateDictionary WScript.Echo WScript.Echo "Certificate type Id: " & Pair.Key WScript.Echo "Certificate: " & Pair.Value Next
# Shows how to obtain a new application certificate pack from the certificate manager (GDS), and store it for subsequent # usage. # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . # OPC client and subscriber examples in Python on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-Python . # Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own # a commercial license in order to use Online Forums, and we reply to every post. # The QuickOPC package is needed. Install it using "pip install opclabs_quickopc". import opclabs_quickopc # Import .NET namespaces. from OpcLabs.EasyOpc.UA import * from OpcLabs.EasyOpc.UA.Application import * from OpcLabs.EasyOpc.UA.Application.Extensions import * from OpcLabs.EasyOpc.UA.Extensions import * from OpcLabs.EasyOpc.UA.OperationModel import * # Define which GDS we will work with. gdsEndpointDescriptor = UAEndpointDescriptor('opc.tcp://opcua.demo-this.com:58810/GlobalDiscoveryServer') gdsEndpointDescriptor = UAEndpointDescriptorExtension.WithUserNameIdentity(gdsEndpointDescriptor, 'appadmin', 'demo') # Obtain the application interface. application = EasyUAApplication.Instance # Display which application we are about to work with. applicationElement = IEasyUAClientServerApplicationExtension.GetApplicationElement(application) print('Application URI string: ', applicationElement.ApplicationUriString, sep='') # Obtain a new application certificate pack from the certificate manager (GDS), and store it for subsequent usage. try: print('Obtaining new certificate pack...') certificateDictionary = IEasyUAClientServerApplicationExtension.ObtainNewCertificatePack(application, gdsEndpointDescriptor) except UAException as uaException: print('*** Failure: ' + uaException.GetBaseException().Message) exit() # Display results. for pair in certificateDictionary: print() print('Certificate type Id: ', pair.Key, sep='') print('Certificate: ', pair.Value, sep='') print() print('Finished.')
// Shows how to refresh own certificate stores using current trust lists for the application from the certificate manager. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in C# on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-CSharp . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. using System; using OpcLabs.EasyOpc.UA; using OpcLabs.EasyOpc.UA.Application; using OpcLabs.EasyOpc.UA.Application.Extensions; using OpcLabs.EasyOpc.UA.Extensions; using OpcLabs.EasyOpc.UA.Gds; using OpcLabs.EasyOpc.UA.OperationModel; namespace UADocExamples.Application._IEasyUAClientServerApplication { class RefreshTrustLists { public static void Main1() { // Define which GDS we will work with. UAEndpointDescriptor gdsEndpointDescriptor = ((UAEndpointDescriptor)"opc.tcp://opcua.demo-this.com:58810/GlobalDiscoveryServer") .WithUserNameIdentity("appadmin", "demo"); // Obtain the application interface. EasyUAApplication application = EasyUAApplication.Instance; // Display which application we are about to work with. Console.WriteLine("Application URI string: {0}", application.GetApplicationElement().ApplicationUriString); // Refresh own certificate stores using current trust lists for the application from the certificate manager. UATrustListMasks refreshedTrustLists; try { refreshedTrustLists = application.RefreshTrustLists(gdsEndpointDescriptor); } catch (UAException uaException) { Console.WriteLine("*** Failure: {0}", uaException.GetBaseException().Message); return; } // Display results Console.WriteLine("Refreshed trust lists: {0}", refreshedTrustLists); } } }
' Shows how to refresh own certificate stores using current trust lists for the application from the certificate manager. ' ' Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . ' OPC client and subscriber examples in VB.NET on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBNET . ' Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own ' a commercial license in order to use Online Forums, and we reply to every post. Imports OpcLabs.EasyOpc.UA Imports OpcLabs.EasyOpc.UA.Application Imports OpcLabs.EasyOpc.UA.Application.Extensions Imports OpcLabs.EasyOpc.UA.Extensions Imports OpcLabs.EasyOpc.UA.Gds Imports OpcLabs.EasyOpc.UA.OperationModel Namespace Application._IEasyUAClientServerApplication Friend Class RefreshTrustLists Public Shared Sub Main1() ' Define which GDS we will work with. Dim gdsEndpointDescriptor As UAEndpointDescriptor = New UAEndpointDescriptor("opc.tcp://opcua.demo-this.com:58810/GlobalDiscoveryServer") _ .WithUserNameIdentity("appadmin", "demo") ' Obtain the application interface. Dim application As EasyUAApplication = EasyUAApplication.Instance ' Display which application we are about to work with. Console.WriteLine("Application URI string: {0}", application.GetApplicationElement().ApplicationUriString) ' Refresh own certificate stores using current trust lists for the application from the certificate manager. Dim refreshedTrustLists As UATrustListMasks Try refreshedTrustLists = application.RefreshTrustLists(gdsEndpointDescriptor) Catch uaException As UAException Console.WriteLine("*** Failure: {0}", uaException.GetBaseException.Message) Exit Sub End Try ' Display results Console.WriteLine("Refreshed trust lists: {0}", refreshedTrustLists) End Sub End Class End Namespace
// Shows how to refresh own certificate stores using current trust lists // for the application from the certificate manager. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in Object Pascal (Delphi) on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-OP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. class procedure RefreshTrustLists.Main; var Application: TEasyUAApplication; GdsEndpointDescriptor: _UAEndpointDescriptor; RefreshedTrustLists: UATrustListMasks; begin // Define which GDS we will work with. GdsEndpointDescriptor := CoUAEndpointDescriptor.Create; GdsEndpointDescriptor.UrlString := 'opc.tcp://opcua.demo-this.com:58810/GlobalDiscoveryServer'; GdsEndpointDescriptor.UserIdentity.UserNameTokenInfo.UserName := 'appadmin'; GdsEndpointDescriptor.UserIdentity.UserNameTokenInfo.Password := 'demo'; // Obtain the application interface. Application := TEasyUAApplication.Create(nil); // Display which application we are about to work with. WriteLn('Application URI string: ', Application.GetApplicationElement.ApplicationUriString); // Refresh own certificate stores using current trust lists for the application from the certificate manager. try RefreshedTrustLists := Application.RefreshTrustLists(gdsEndpointDescriptor, True); except on E: EOleException do begin WriteLn(Format('*** Failure: %s', [E.GetBaseException.Message])); Exit; end; end; // Display results WriteLn('Refreshed trust lists: ', RefreshedTrustLists); end;
// Shows how to refresh own certificate stores using current trust lists // for the application from the certificate manager. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in PHP on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-PHP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. // Define which GDS we will work with. $GdsEndpointDescriptor = new COM("OpcLabs.EasyOpc.UA.UAEndpointDescriptor"); $GdsEndpointDescriptor->UrlString = "opc.tcp://opcua.demo-this.com:58810/GlobalDiscoveryServer"; $GdsEndpointDescriptor->UserIdentity->UserNameTokenInfo->UserName = "appadmin"; $GdsEndpointDescriptor->UserIdentity->UserNameTokenInfo->Password = "demo"; // Obtain the application interface. $Application = new COM("OpcLabs.EasyOpc.UA.Application.EasyUAApplication"); // Display which application we are about to work with. printf("Application URI string: %s\n", $Application->GetApplicationElement->ApplicationUriString); // Refresh own certificate stores using current trust lists for the application from the certificate manager. try { $RefreshedTrustLists = $Application->RefreshTrustLists($GdsEndpointDescriptor, true); } catch (com_exception $e) { printf("*** Failure: %s\n", $e->getMessage()); exit(); } // Display results printf("Refreshed trust lists: %s\n", $RefreshedTrustLists);
REM Shows how to refresh own certificate stores using current trust lists REM for the application from the certificate manager. REM REM Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . REM OPC client and subscriber examples in Visual Basic on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VB . REM Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own REM a commercial license in order to use Online Forums, and we reply to every post. Private Sub IEasyUAClientServerApplication_RefreshTrustLists_Main_Command_Click() OutputText = "" ' Define which GDS we will work with. Dim gdsEndpointDescriptor As New UAEndpointDescriptor gdsEndpointDescriptor.UrlString = "opc.tcp://opcua.demo-this.com:58810/GlobalDiscoveryServer" gdsEndpointDescriptor.UserIdentity.UserNameTokenInfo.UserName = "appadmin" gdsEndpointDescriptor.UserIdentity.UserNameTokenInfo.Password = "demo" ' Obtain the application interface Dim Application As New EasyUAApplication ' Display which application we are about to work with. OutputText = OutputText & "Application URI string: " & Application.GetApplicationElement.applicationUriString & vbCrLf ' Refresh own certificate stores using current trust lists for the application from the certificate manager. On Error Resume Next Dim refreshedTrustLists As UATrustListMasks refreshedTrustLists = Application.RefreshTrustLists(gdsEndpointDescriptor, True) If Err.Number <> 0 Then OutputText = OutputText & "*** Failure: " & Err.Source & ": " & Err.Description & vbCrLf Exit Sub End If On Error GoTo 0 ' Display results OutputText = OutputText & "Refreshed trust lists: " & refreshedTrustLists & vbCrLf End Sub
# Shows how to refresh own certificate stores using current trust lists for the application from the certificate # manager. # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . # OPC client and subscriber examples in Python on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-Python . # Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own # a commercial license in order to use Online Forums, and we reply to every post. # The QuickOPC package is needed. Install it using "pip install opclabs_quickopc". import opclabs_quickopc # Import .NET namespaces. from System import * from OpcLabs.EasyOpc.UA import * from OpcLabs.EasyOpc.UA.Application import * from OpcLabs.EasyOpc.UA.Application.Extensions import * from OpcLabs.EasyOpc.UA.Extensions import * from OpcLabs.EasyOpc.UA.Gds import * from OpcLabs.EasyOpc.UA.OperationModel import * # Define which GDS we will work with. gdsEndpointDescriptor = UAEndpointDescriptor('opc.tcp://opcua.demo-this.com:58810/GlobalDiscoveryServer') gdsEndpointDescriptor = UAEndpointDescriptorExtension.WithUserNameIdentity(gdsEndpointDescriptor, 'appadmin', 'demo') # Obtain the application interface. application = EasyUAApplication.Instance # Display which application we are about to work with. applicationElement = IEasyUAClientServerApplicationExtension.GetApplicationElement(application) print('Application URI string: ', applicationElement.ApplicationUriString, sep='') # Refresh own certificate stores using current trust lists for the application from the certificate manager. try: print('Refreshing trust lists...') refreshedTrustLists = IEasyUAClientServerApplicationExtension.RefreshTrustLists(application, gdsEndpointDescriptor) except UAException as uaException: print('*** Failure: ' + uaException.GetBaseException().Message) exit() # Display results. print('Refreshed trust lists: ', Enum.Format(UATrustListMasks, refreshedTrustLists, 'G'), sep='') print() print('Finished.')