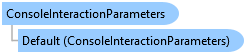
'Declaration
<CLSCompliantAttribute(True)> <ComDefaultInterfaceAttribute(OpcLabs.BaseLib.Console.Interaction.ComTypes._ConsoleInteractionParameters)> <ComVisibleAttribute(True)> <GuidAttribute("CCD03429-F735-43B8-A023-8E4C5344478A")> <TypeConverterAttribute(System.ComponentModel.ExpandableObjectConverter)> <ValueControlAttribute("OpcLabs.BaseLib.Forms.Common.ObjectSerializationControl, OpcLabs.BaseLibForms, Version=5.81.455.1, Culture=neutral, PublicKeyToken=6faddca41dacb409", DefaultReadWrite=False, Export=True, PageId=10001)> <SerializableAttribute()> Public Class ConsoleInteractionParameters Inherits OpcLabs.BaseLib.Parameters Implements OpcLabs.BaseLib.ComTypes._Info, OpcLabs.BaseLib.ComTypes._Object2, OpcLabs.BaseLib.ComTypes._Parameters, OpcLabs.BaseLib.Console.Interaction.ComTypes._ConsoleInteractionParameters, System.ICloneable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
'Usage
Dim instance As ConsoleInteractionParameters
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.BaseLib.Console.Interaction.ComTypes._ConsoleInteractionParameters)] [ComVisible(true)] [Guid("CCD03429-F735-43B8-A023-8E4C5344478A")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [ValueControl("OpcLabs.BaseLib.Forms.Common.ObjectSerializationControl, OpcLabs.BaseLibForms, Version=5.81.455.1, Culture=neutral, PublicKeyToken=6faddca41dacb409", DefaultReadWrite=false, Export=true, PageId=10001)] [Serializable()] public class ConsoleInteractionParameters : OpcLabs.BaseLib.Parameters, OpcLabs.BaseLib.ComTypes._Info, OpcLabs.BaseLib.ComTypes._Object2, OpcLabs.BaseLib.ComTypes._Parameters, OpcLabs.BaseLib.Console.Interaction.ComTypes._ConsoleInteractionParameters, System.ICloneable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.BaseLib.Console.Interaction.ComTypes._ConsoleInteractionParameters)] [ComVisible(true)] [Guid("CCD03429-F735-43B8-A023-8E4C5344478A")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [ValueControl("OpcLabs.BaseLib.Forms.Common.ObjectSerializationControl, OpcLabs.BaseLibForms, Version=5.81.455.1, Culture=neutral, PublicKeyToken=6faddca41dacb409", DefaultReadWrite=false, Export=true, PageId=10001)] [Serializable()] public ref class ConsoleInteractionParameters : public OpcLabs.BaseLib.Parameters, OpcLabs.BaseLib.ComTypes._Info, OpcLabs.BaseLib.ComTypes._Object2, OpcLabs.BaseLib.ComTypes._Parameters, OpcLabs.BaseLib.Console.Interaction.ComTypes._ConsoleInteractionParameters, System.ICloneable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
// Shows how to configure the OPC UA Console Interaction plug-in by turning off the output colorization. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in C# on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-CSharp . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. using System; using System.Diagnostics; using OpcLabs.BaseLib.Console.Interaction; using OpcLabs.EasyOpc.UA; using OpcLabs.EasyOpc.UA.Engine; using OpcLabs.EasyOpc.UA.OperationModel; namespace UADocExamples.Interaction { partial class ConsoleInteraction { public static void ColorizeOutput() { // Configure the shared plug-in. // Find the parameters object of the plug-in. ConsoleInteractionParameters consoleInteractionPluginParameters = EasyUAClient.SharedParameters.PluginConfigurations.Find<ConsoleInteractionParameters>(); Debug.Assert(consoleInteractionPluginParameters != null); // Change the parameter. consoleInteractionPluginParameters.ColorizeOutput = false; // Do not implicitly trust any endpoint URLs. We want the user be asked explicitly. EasyUAClient.SharedParameters.EngineParameters.CertificateAcceptancePolicy.TrustedEndpointUrlStrings.Clear(); // Define which server we will work with. UAEndpointDescriptor endpointDescriptor = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer"; // Require secure connection, in order to enforce the certificate check. endpointDescriptor.EndpointSelectionPolicy = new UAEndpointSelectionPolicy(UAMessageSecurityModes.Secure); // Instantiate the client object. var client = new EasyUAClient(); UAAttributeData attributeData; try { // Obtain attribute data. // The component automatically triggers the necessary user interaction during the first operation. attributeData = client.Read(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10853"); } catch (UAException uaException) { Console.WriteLine("*** Failure: {0}", uaException.GetBaseException().Message); return; } // Display results. Console.WriteLine("Value: {0}", attributeData.Value); Console.WriteLine("ServerTimestamp: {0}", attributeData.ServerTimestamp); Console.WriteLine("SourceTimestamp: {0}", attributeData.SourceTimestamp); Console.WriteLine("StatusCode: {0}", attributeData.StatusCode); } } }
' Shows how to configure the OPC UA Console Interaction plug-in by turning off the output colorization. ' ' Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . ' OPC client and subscriber examples in VB.NET on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBNET . ' Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own ' a commercial license in order to use Online Forums, and we reply to every post. Imports OpcLabs.BaseLib.Console.Interaction Imports OpcLabs.EasyOpc.UA Imports OpcLabs.EasyOpc.UA.Engine Imports OpcLabs.EasyOpc.UA.OperationModel Namespace Interaction Friend Class ConsoleInteraction Public Shared Sub ColorizeOutput() ' Configure the shared plug-in. ' Find the parameters object of the plug-in. Dim consoleInteractionPluginParameters = EasyUAClient.SharedParameters.PluginConfigurations.Find(Of ConsoleInteractionParameters)() Debug.Assert(consoleInteractionPluginParameters IsNot Nothing) ' Change the parameter. consoleInteractionPluginParameters.ColorizeOutput = False ' Do not implicitly trust any endpoint URLs. We want the user be asked explicitly. EasyUAClient.SharedParameters.EngineParameters.CertificateAcceptancePolicy.TrustedEndpointUrlStrings.Clear() ' Define which server we will work with. Dim endpointDescriptor As UAEndpointDescriptor = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" ' Require secure connection, in order to enforce the certificate check. endpointDescriptor.EndpointSelectionPolicy = New UAEndpointSelectionPolicy(UAMessageSecurityModes.Secure) ' Instantiate the client object. Dim client = New EasyUAClient() Dim attributeData As UAAttributeData Try ' Obtain attribute data. ' The component automatically triggers the necessary user interaction during the first operation. attributeData = client.Read(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10853") Catch uaException As UAException Console.WriteLine("*** Failure: {0}", uaException.GetBaseException.Message) Exit Sub End Try ' Display results. Console.WriteLine("Value: {0}", attributeData.Value) Console.WriteLine("ServerTimestamp: {0}", attributeData.ServerTimestamp) Console.WriteLine("SourceTimestamp: {0}", attributeData.SourceTimestamp) Console.WriteLine("StatusCode: {0}", attributeData.StatusCode) End Sub End Class End Namespace
// Shows how to configure the OPC UA Console Interaction plug-in by turning off the output colorization. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in Object Pascal (Delphi) on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-OP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. class procedure ConsoleInteraction.ColorizeOutput; var Arguments: OleVariant; AttributeData: _UAAttributeData; Client: OpcLabs_EasyOpcUA_TLB._EasyUAClient; ClientManagement: TEasyUAClientManagement; ConsoleInteractionParameters: _ConsoleInteractionParameters; EndpointSelectionPolicy: _UAEndpointSelectionPolicy; ReadArguments: _UAReadArguments; Result: _UAAttributeDataResult; Results: OleVariant; begin // Configure the shared plug-in. // The configuration object allows access to static behavior. ClientManagement := TEasyUAClientManagement.Create(nil); ClientManagement.Connect; // Find the parameters object of the plug-in. ConsoleInteractionParameters := IUnknown(ClientManagement.SharedParameters.PluginConfigurations.Find('OpcLabs.BaseLib.Console.Interaction.ConsoleInteractionParameters')) as _ConsoleInteractionParameters; // Change the parameter. ConsoleInteractionParameters.ColorizeOutput := False; // Do not implicitly trust any endpoint URLs. We want the user be asked explicitly. ClientManagement.SharedParameters.EngineParameters.CertificateAcceptancePolicy.TrustedEndpointUrlStrings.Clear(); // Define which server we will work with. ReadArguments := CoUAReadArguments.Create; ReadArguments.EndpointDescriptor.UrlString := 'opc.tcp://opcua.demo-this.com:51210/UA/SampleServer'; // Require secure connection, in order to enforce the certificate check. EndpointSelectionPolicy := CoUAEndpointSelectionPolicy.Create; EndpointSelectionPolicy.AllowedMessageSecurityModes := UAMessageSecurityModes_Secure; ReadArguments.EndpointDescriptor.EndpointSelectionPolicy := EndpointSelectionPolicy; ReadArguments.NodeDescriptor.NodeId.ExpandedText := 'nsu=http://test.org/UA/Data/ ;i=10853'; Arguments := VarArrayCreate([0, 0], varVariant); Arguments[0] := ReadArguments; // Instantiate the client object. Client := CoEasyUAClient.Create; // Obtain attribute data. // The component automatically triggers the necessary user interaction during the first operation. TVarData(Results).VType := varArray or varVariant; TVarData(Results).VArray := PVarArray(Client.ReadMultiple(Arguments)); Result := IInterface(Results[0]) as _UAAttributeDataResult; if Result.Succeeded then begin AttributeData := Result.AttributeData; // Display results. WriteLn('Value: ', AttributeData.Value); WriteLn('ServerTimestamp: ', DateTimeToStr(AttributeData.ServerTimestamp)); WriteLn('SourceTimestamp: ', DateTimeToStr(AttributeData.SourceTimestamp)); WriteLn('StatusCode: ', AttributeData.StatusCode.ToString); end else WriteLn('*** Failure: ', Result.ErrorMessageBrief); VarClear(Results); VarClear(Arguments); FreeAndNil(ClientManagement); end;
# Shows how to configure the OPC UA Console Interaction plug-in by turning off the output colorization. # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . # OPC client and subscriber examples in Python on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-Python . # Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own # a commercial license in order to use Online Forums, and we reply to every post. # The QuickOPC package is needed. Install it using "pip install opclabs_quickopc". import opclabs_quickopc # Import .NET namespaces. from OpcLabs.BaseLib.Console.Interaction import * from OpcLabs.EasyOpc.UA import * from OpcLabs.EasyOpc.UA.Engine import * from OpcLabs.EasyOpc.UA.OperationModel import * # Configure the shared plug-in. # Find the parameters object of the plug-in. consoleInteractionPluginParameters = EasyUAClient.SharedParameters.PluginConfigurations.Find[ConsoleInteractionParameters]() assert consoleInteractionPluginParameters is not None # Change the parameter. consoleInteractionPluginParameters.ColorizeOutput = False # Do not implicitly trust any endpoint URLs. We want the user be asked explicitly. EasyUAClient.SharedParameters.EngineParameters.CertificateAcceptancePolicy.TrustedEndpointUrlStrings.Clear() # Define which server we will work with. endpointDescriptor = UAEndpointDescriptor('opc.tcp://opcua.demo-this.com:51210/UA/SampleServer') # Require secure connection, in order to enforce the certificate check. endpointDescriptor.EndpointSelectionPolicy = UAEndpointSelectionPolicy(UAMessageSecurityModes.Secure) # Instantiate the client object. client = EasyUAClient() try: # Obtain attribute data. # The component automatically triggers the necessary user interaction during the first operation. attributeData = IEasyUAClientExtension.Read(client, endpointDescriptor, UANodeDescriptor('nsu=http://test.org/UA/Data/ ;i=10853')) except UAException as uaException: print('*** Failure: ' + uaException.GetBaseException().Message) exit() # Display results. print('Value: ', attributeData.Value) print('ServerTimestamp: ', attributeData.ServerTimestamp) print('SourceTimestamp: ', attributeData.SourceTimestamp) print('StatusCode: ', attributeData.StatusCode) print() print('Finished.')
System.Object
OpcLabs.BaseLib.Object2
OpcLabs.BaseLib.Info
OpcLabs.BaseLib.Parameters
OpcLabs.BaseLib.Console.Interaction.ConsoleInteractionParameters