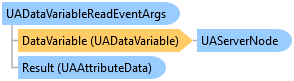
'Declaration
<ExceptionContractAnnotationAttribute(True)> <CLSCompliantAttribute(True)> Public Class UADataVariableReadEventArgs Inherits UADataVariableProcessedEventArgs
'Usage
Dim instance As UADataVariableReadEventArgs
[ExceptionContractAnnotation(true)] [CLSCompliant(true)] public class UADataVariableReadEventArgs : UADataVariableProcessedEventArgs
[ExceptionContractAnnotation(true)] [CLSCompliant(true)] public ref class UADataVariableReadEventArgs : public UADataVariableProcessedEventArgs
// This example shows how to handle the read event on a folder, providing a way to implement reading of multiple data // variables using a single handler. // You can use any OPC UA client, including our Connectivity Explorer and OpcCmd utility, to connect to the server. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client, server and subscriber examples in C# on GitHub: https://github.com/OPCLabs/Examples-OPCStudio-CSharp . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. using System; using OpcLabs.BaseLib.NodeSpace; using OpcLabs.EasyOpc.UA; using OpcLabs.EasyOpc.UA.NodeSpace; using OpcLabs.EasyOpc.UA.OperationModel; namespace UAServerDocExamples._UAServerNode { class Read { public static void Main1() { // Instantiate the server object. // By default, the server will run on endpoint URL "opc.tcp://localhost:48040/". var server = new EasyUAServer(); // Create a folder node. UAFolder myFolder = UAFolder.CreateIn(server.Objects, "MyFolder"); // Create data variables in the folder. Distinguish them by their state. myFolder.Add(new UADataVariable("MyDataVariable1").ValueType<int>().SetState(1)); myFolder.Add(new UADataVariable("MyDataVariable2").ValueType<int>().SetState(2)); myFolder.Add(new UADataVariable("MyDataVariable3").ValueType<int>().SetState(3)); myFolder.Add(new UADataVariable("MyDataVariable4").ValueType<int>().SetState(4)); myFolder.Add(new UADataVariable("MyDataVariable5").ValueType<int>().SetState(5)); // Handle the read event for the folder. myFolder.Read += MyFolderOnRead; // Start the server. Console.WriteLine("The server is starting..."); server.Start(); Console.WriteLine("The server is started."); Console.WriteLine(); // Let the user decide when to stop. Console.WriteLine("Press Enter to stop the server..."); Console.ReadLine(); // Stop the server. Console.WriteLine("The server is stopping..."); server.Stop(); Console.WriteLine("The server is stopped."); } /// <summary> /// Event handler for the read event on the folder. /// </summary> /// <param name="sender">The folder object that sends the event.</param> /// <param name="e">Data for the variable read event.</param> static private void MyFolderOnRead(object sender, UADataVariableReadEventArgs e) { // Obtain the state associated with the data variable that is being read. // Use it as the offset for the random value, so that each data variable generates values in a unique range. int offset = (int)e.DataVariable.State*100; // Generate a random value, indicate that the read has been handled, and return the generated value. e.HandleAndReturn(Random.Next(offset, offset + 100)); } static private readonly Random Random = new Random(); } }
' This example shows how to handle the read event on a folder, providing a way to implement reading of multiple data ' variables using a single handler. ' You can use any OPC UA client, including our Connectivity Explorer and OpcCmd utility, to connect to the server. ' ' Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . ' OPC client and subscriber examples in VB.NET on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBNET . ' Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own ' a commercial license in order to use Online Forums, and we reply to every post. Imports System Imports OpcLabs.BaseLib.NodeSpace Imports OpcLabs.EasyOpc.UA Imports OpcLabs.EasyOpc.UA.NodeSpace Imports OpcLabs.EasyOpc.UA.OperationModel Namespace _UAServerNode Partial Friend Class Read Shared Sub Main1() ' Instantiate the server object. ' By default, the server will run on endpoint URL "opc.tcp://localhost:48040/". Dim server = New EasyUAServer() ' Create a folder node. Dim myFolder As UAFolder = UAFolder.CreateIn(server.Objects, "MyFolder") ' Create data variables in the folder. Distinguish them by their state. myFolder.Add(New UADataVariable("MyDataVariable1").ValueType(Of Integer)().SetState(1)) myFolder.Add(New UADataVariable("MyDataVariable2").ValueType(Of Integer)().SetState(2)) myFolder.Add(New UADataVariable("MyDataVariable3").ValueType(Of Integer)().SetState(3)) myFolder.Add(New UADataVariable("MyDataVariable4").ValueType(Of Integer)().SetState(4)) myFolder.Add(New UADataVariable("MyDataVariable5").ValueType(Of Integer)().SetState(5)) ' Handle the read event for the folder. AddHandler myFolder.Read, AddressOf MyFolderOnRead ' Start the server. Console.WriteLine("The server is starting...") server.Start() Console.WriteLine("The server is started.") Console.WriteLine() ' Let the user decide when to stop. Console.WriteLine("Press Enter to stop the server...") Console.ReadLine() ' Stop the server. Console.WriteLine("The server is stopping...") server.Stop() Console.WriteLine("The server is stopped.") End Sub ''' <summary> ''' Event handler for the read event on the folder. ''' </summary> ''' <param name="sender">The data variable object that sends the event.</param> ''' <param name="e">Data for the variable read event.</param> Private Shared Sub MyFolderOnRead(ByVal sender As Object, ByVal e As UADataVariableReadEventArgs) ' Obtain the state associated with the data variable that is being read. ' Use it as the offset for the random value, so that each data variable generates values in a unique range. Dim offset As Integer = CInt(e.DataVariable.State * 100) ' Generate a random value, indicate that the read has been handled, and return the generated value. e.HandleAndReturn(Random.Next(offset, offset + 100)) End Sub Private Shared ReadOnly Random As Random = New Random() End Class End Namespace
System.Object
System.EventArgs
System.ComponentModel.HandledEventArgs
OpcLabs.BaseLib.Widgets.ProcessedEventArgs
OpcLabs.EasyOpc.UA.OperationModel.UADataVariableProcessedEventArgs
OpcLabs.EasyOpc.UA.OperationModel.UADataVariableReadEventArgs