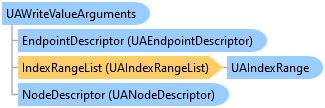
'Declaration
<CLSCompliantAttribute(True)> <ComDefaultInterfaceAttribute(OpcLabs.EasyOpc.UA.OperationModel.ComTypes._UAWriteValueArguments)> <ComVisibleAttribute(True)> <GuidAttribute("17F223BC-DEE0-4657-9AC7-A94F56E6A29D")> <TypeConverterAttribute(System.ComponentModel.ExpandableObjectConverter)> <ValueControlAttribute("OpcLabs.BaseLib.Forms.Common.ObjectSerializationControl, OpcLabs.BaseLibForms, Version=5.81.455.1, Culture=neutral, PublicKeyToken=6faddca41dacb409", DefaultReadWrite=False, Export=True, PageId=10001)> <SerializableAttribute()> Public Class UAWriteValueArguments Inherits UAWriteArgumentsBase Implements LINQPad.ICustomMemberProvider, OpcLabs.BaseLib.ComTypes._Info, OpcLabs.BaseLib.ComTypes._Object2, OpcLabs.BaseLib.OperationModel.ComTypes._OperationArguments, OpcLabs.EasyOpc.UA.OperationModel.ComTypes._UAAttributeArguments, OpcLabs.EasyOpc.UA.OperationModel.ComTypes._UANodeArguments, OpcLabs.EasyOpc.UA.OperationModel.ComTypes._UAWriteArgumentsBase, OpcLabs.EasyOpc.UA.OperationModel.ComTypes._UAWriteValueArguments, System.ICloneable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
'Usage
Dim instance As UAWriteValueArguments
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.EasyOpc.UA.OperationModel.ComTypes._UAWriteValueArguments)] [ComVisible(true)] [Guid("17F223BC-DEE0-4657-9AC7-A94F56E6A29D")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [ValueControl("OpcLabs.BaseLib.Forms.Common.ObjectSerializationControl, OpcLabs.BaseLibForms, Version=5.81.455.1, Culture=neutral, PublicKeyToken=6faddca41dacb409", DefaultReadWrite=false, Export=true, PageId=10001)] [Serializable()] public class UAWriteValueArguments : UAWriteArgumentsBase, LINQPad.ICustomMemberProvider, OpcLabs.BaseLib.ComTypes._Info, OpcLabs.BaseLib.ComTypes._Object2, OpcLabs.BaseLib.OperationModel.ComTypes._OperationArguments, OpcLabs.EasyOpc.UA.OperationModel.ComTypes._UAAttributeArguments, OpcLabs.EasyOpc.UA.OperationModel.ComTypes._UANodeArguments, OpcLabs.EasyOpc.UA.OperationModel.ComTypes._UAWriteArgumentsBase, OpcLabs.EasyOpc.UA.OperationModel.ComTypes._UAWriteValueArguments, System.ICloneable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.EasyOpc.UA.OperationModel.ComTypes._UAWriteValueArguments)] [ComVisible(true)] [Guid("17F223BC-DEE0-4657-9AC7-A94F56E6A29D")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [ValueControl("OpcLabs.BaseLib.Forms.Common.ObjectSerializationControl, OpcLabs.BaseLibForms, Version=5.81.455.1, Culture=neutral, PublicKeyToken=6faddca41dacb409", DefaultReadWrite=false, Export=true, PageId=10001)] [Serializable()] public ref class UAWriteValueArguments : public UAWriteArgumentsBase, LINQPad.ICustomMemberProvider, OpcLabs.BaseLib.ComTypes._Info, OpcLabs.BaseLib.ComTypes._Object2, OpcLabs.BaseLib.OperationModel.ComTypes._OperationArguments, OpcLabs.EasyOpc.UA.OperationModel.ComTypes._UAAttributeArguments, OpcLabs.EasyOpc.UA.OperationModel.ComTypes._UANodeArguments, OpcLabs.EasyOpc.UA.OperationModel.ComTypes._UAWriteArgumentsBase, OpcLabs.EasyOpc.UA.OperationModel.ComTypes._UAWriteValueArguments, System.ICloneable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
If you want to write a data value into an attribute of a specific node in OPC UA, call the WriteValue method, passing it the data value you want to write, arguments for endpoint descriptor, node ID, and an optional data type.
When you specify individual arguments to the simplest overload of the WriteValue method, the method will write to the Value attribute of the node, and if the value type is an array, it will modify the whole contents of that array. If you want to write into a different attribute, or write only to a subset of an array, use one of the more complex overloads of the WriteValue method, such as with an argument of UAWriteValueArguments type, and fill this argument will all necessary information.
For writing data values into multiple OPC attributes (in the same or different nodes) in an efficient manner, call the WriteMultipleValues method. You pass in an array of UAWriteValueArgument objects, each specifying the location of OPC node, attribute, and the value to be written.
The example above can be extended by properly testing for success of each operation, like this:
The WriteMultiple and WriteMultipleValues methods return an array of UAWriteResult objects. Besides the inherited members of OperationResult, such as the Exception, the UAWriteResult contains additional information that describes the outcome of the Write operation in case of success:
If you access some node or nodes repeatedly, it might be possible to improve the performance of it by (pre-)registering the node or nodes with the server. The performance improvement will only occur if the target OPC UA server supports the necessary node registration services. For more information, see OPC UA Node Registration Service.
// This example shows how to write values into 3 nodes at once, test for success of each write and display the exception // message in case of failure. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in C# on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-CSharp . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. using System; using OpcLabs.BaseLib.OperationModel; using OpcLabs.EasyOpc.UA; using OpcLabs.EasyOpc.UA.OperationModel; namespace UADocExamples._EasyUAClient { partial class WriteMultipleValues { public static void TestSuccess() { UAEndpointDescriptor endpointDescriptor = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer"; // or "http://opcua.demo-this.com:51211/UA/SampleServer" (currently not supported) // or "https://opcua.demo-this.com:51212/UA/SampleServer/" // Instantiate the client object. var client = new EasyUAClient(); Console.WriteLine("Modifying values of nodes..."); OperationResult[] operationResultArray = client.WriteMultipleValues(new[] { new UAWriteValueArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10221", 23456), new UAWriteValueArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10226", "This string cannot be converted to Double"), new UAWriteValueArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;s=UnknownNode", "ABC") }); for (int i = 0; i < operationResultArray.Length; i++) if (operationResultArray[i].Succeeded) Console.WriteLine($"Result {i}: success"); else Console.WriteLine($"Result {i}: {operationResultArray[i].Exception.GetBaseException().Message}"); // Example output: // //Modifying values of nodes... //Result 0: success //Result 1: Input string was not in a correct format. //+ Attempting to change an object of type "System.String" to type "System.Double". //+ The specified original value (string) was "This string cannot be converted to Double". //+ The node descriptor used was: NodeId="nsu=http://test.org/UA/Data/ ;i=10226". //+ The client method called (or event/callback invoked) was 'WriteMultiple[3]'. //Result 2: The status of the OPC-UA attribute data is not Good. The actual status is 'BadNodeIdUnknown'. //+ During writing or method calls, readings may occur when value type is not specified. //+ The node descriptor used was: NodeId="nsu=http://test.org/UA/Data/ ;s=UnknownNode". //+ The client method called (or event/callback invoked) was 'WriteMultiple[3]'. } } }
# This example shows how to write values into 3 nodes at once, test for success of each write and display the exception # message in case of failure. # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . # OPC client and subscriber examples in PowerShell on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-PowerShell . # Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own # a commercial license in order to use Online Forums, and we reply to every post. #requires -Version 5.1 using namespace OpcLabs.EasyOpc.UA using namespace OpcLabs.EasyOpc.UA.OperationModel # The path below assumes that the current directory is [ProductDir]/Examples-NET/PowerShell/Windows . Add-Type -Path "../../../Components/Opclabs.QuickOpc/net472/OpcLabs.EasyOpcUA.dll" Add-Type -Path "../../../Components/Opclabs.QuickOpc/net472/OpcLabs.EasyOpcUAComponents.dll" [UAEndpointDescriptor]$endpointDescriptor = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" # or "http://opcua.demo-this.com:51211/UA/SampleServer" (currently not supported) # or "https://opcua.demo-this.com:51212/UA/SampleServer/" # Instantiate the client object. $client = New-Object EasyUAClient Write-Host "Modifying values of nodes..." $operationResultArray = $client.WriteMultipleValues([UAWriteValueArguments[]]@( (New-Object UAWriteValueArguments($endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10221", 23456)), (New-Object UAWriteValueArguments($endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10226", "This string cannot be converted to Double")), (New-Object UAWriteValueArguments($endpointDescriptor, "nsu=http://test.org/UA/Data/ ;s=UnknownNode", "ABC")) )) for ($i = 0; $i -lt $operationResultArray.Length; $i++) { if ($operationResultArray[$i].Succeeded) { Write-Host "Result $($i): success" } else { Write-Host "Result $($i): $($operationResultArray[$i].Exception.GetBaseException().Message)" } } # Example output: # #Modifying values of nodes... #Result 0: success #Result 1: Input string was not in a correct format. #+ Attempting to change an object of type "System.String" to type "System.Double". #+ The specified original value (string) was "This string cannot be converted to Double". #+ The node descriptor used was: NodeId="nsu=http://test.org/UA/Data/ ;i=10226". #+ The client method called (or event/callback invoked) was 'WriteMultiple[3]'. #Result 2: The status of the OPC-UA attribute data is not Good. The actual status is 'BadNodeIdUnknown'. #+ During writing or method calls, readings may occur when value type is not specified. #+ The node descriptor used was: NodeId="nsu=http://test.org/UA/Data/ ;s=UnknownNode". #+ The client method called (or event/callback invoked) was 'WriteMultiple[3]'.
' This example shows how to write values into 3 nodes at once, test for success of each write and display the exception ' message in case of failure. ' ' Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . ' OPC client and subscriber examples in VB.NET on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBNET . ' Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own ' a commercial license in order to use Online Forums, and we reply to every post. Imports System Imports OpcLabs.BaseLib.OperationModel Imports OpcLabs.EasyOpc.UA Imports OpcLabs.EasyOpc.UA.OperationModel Namespace _EasyUAClient Partial Friend Class WriteMultipleValues Public Shared Sub TestSuccess() ' Define which server we will work with. Dim endpointDescriptor As UAEndpointDescriptor = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" ' or "http://opcua.demo-this.com:51211/UA/SampleServer" (currently not supported) ' or "https://opcua.demo-this.com:51212/UA/SampleServer/" ' Instantiate the client object Dim client = New EasyUAClient() ' Modify value of a node Dim operationResultArray() As OperationResult = client.WriteMultipleValues(New UAWriteValueArguments() _ { _ New UAWriteValueArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10221", 23456), _ New UAWriteValueArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10226", "This string cannot be converted to Double"), _ New UAWriteValueArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;s=UnknownNode", "ABC") _ } _ ) For i As Integer = 0 To operationResultArray.Length - 1 If operationResultArray(i).Succeeded Then Console.WriteLine("Result {0}: success", i) Else Console.WriteLine("Result {0}: {1}", i, operationResultArray(i).Exception.GetBaseException().Message) End If Next i End Sub End Class End Namespace
# This example shows how to write values into 3 nodes at once, test for success of each write and display the exception # message in case of failure. # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . # OPC client and subscriber examples in Python on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-Python . # Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own # a commercial license in order to use Online Forums, and we reply to every post. # The QuickOPC package is needed. Install it using "pip install opclabs_quickopc". import opclabs_quickopc # Import .NET namespaces. from OpcLabs.EasyOpc.UA import * from OpcLabs.EasyOpc.UA.OperationModel import * endpointDescriptor = UAEndpointDescriptor('opc.tcp://opcua.demo-this.com:51210/UA/SampleServer') # or 'http://opcua.demo-this.com:51211/UA/SampleServer' (currently not supported) # or 'https://opcua.demo-this.com:51212/UA/SampleServer/' # Instantiate the client object client = EasyUAClient() print('Modifying values of nodes...') writeResultArray = IEasyUAClientExtension.WriteMultipleValues(client, [ UAWriteValueArguments(endpointDescriptor, UANodeDescriptor('nsu=http://test.org/UA/Data/ ;i=10221'), 23456), UAWriteValueArguments(endpointDescriptor, UANodeDescriptor('nsu=http://test.org/UA/Data/ ;i=10226'), 'This string cannot be converted to Double'), UAWriteValueArguments(endpointDescriptor, UANodeDescriptor('nsu=http://test.org/UA/Data/ ;s=UnknownNode'), 'ABC'), ]) for i, writeResult in enumerate(writeResultArray): if writeResult.Succeeded: print('writeResultArray[', i, ']: success', sep='') else: print('writeResultArray[', i, '] *** Failure: ', writeResult.Exception.GetBaseException().Message, sep='') print() print('Finished.')
System.Object
OpcLabs.BaseLib.Object2
OpcLabs.BaseLib.Info
OpcLabs.BaseLib.OperationModel.OperationArguments
OpcLabs.EasyOpc.UA.OperationModel.UANodeArguments
OpcLabs.EasyOpc.UA.OperationModel.UAAttributeArguments
OpcLabs.EasyOpc.UA.OperationModel.UAWriteArgumentsBase
OpcLabs.EasyOpc.UA.OperationModel.UAWriteValueArguments