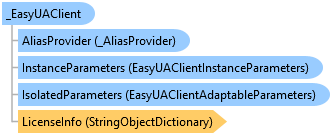
'Declaration
<CLSCompliantAttribute(False)> <ComVisibleAttribute(True)> <GuidAttribute("910344C0-F2A0-42D2-A65E-F3800437F52A")> <InterfaceTypeAttribute(ComInterfaceType.InterfaceIsDual)> Public Interface _EasyUAClient
'Usage
Dim instance As _EasyUAClient
[CLSCompliant(false)] [ComVisible(true)] [Guid("910344C0-F2A0-42D2-A65E-F3800437F52A")] [InterfaceType(ComInterfaceType.InterfaceIsDual)] public interface _EasyUAClient
[CLSCompliant(false)] [ComVisible(true)] [Guid("910344C0-F2A0-42D2-A65E-F3800437F52A")] [InterfaceType(ComInterfaceType.InterfaceIsDual)] public interface class _EasyUAClient
This member or type is for use from COM. It is not meant to be used from .NET or Python. Refer to the corresponding .NET member or type instead, if you are developing in .NET or Python.
// This example shows how to read value of a single node, and display it. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. #include "stdafx.h" // Includes "QuickOpc.h", and other commonly used files #include "ReadValue.h" namespace _EasyUAClient { void ReadValue::Main() { // Initialize the COM library CoInitializeEx(NULL, COINIT_MULTITHREADED); { // Instantiate the client object _EasyUAClientPtr ClientPtr(__uuidof(EasyUAClient)); // Perform the operation _variant_t value = ClientPtr->ReadValue( //L"http://opcua.demo-this.com:51211/UA/SampleServer", L"opc.tcp://opcua.demo-this.com:51210/UA/SampleServer", L"nsu=http://test.org/UA/Data/ ;i=10853"); // Display results _variant_t vString; vString.ChangeType(VT_BSTR, &value); _tprintf(_T("value: %s\n"), (LPCTSTR)CW2CT((_bstr_t)vString)); } // Release all interface pointers BEFORE calling CoUninitialize() CoUninitialize(); } }
// This example shows how to read value of a single node, and display it. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. var Client = new ActiveXObject("OpcLabs.EasyOpc.UA.EasyUAClient"); var value = Client.ReadValue( //"http://opcua.demo-this.com:51211/UA/SampleServer", "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer", "nsu=http://test.org/UA/Data/ ;i=10853"); WScript.Echo("value: " + value);
// This example shows how to read value of a single node, and display it. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. class procedure ReadValue.Main; var Client: EasyUAClient; Value: OleVariant; begin // Instantiate the client object Client := CoEasyUAClient.Create; Value := Client.ReadValue( //'http://opcua.demo-this.com:51211/UA/SampleServer', 'opc.tcp://opcua.demo-this.com:51210/UA/SampleServer', 'nsu=http://test.org/UA/Data/ ;i=10853'); WriteLn('value: ', Value); end;
// This example shows how to read value of a single node, and display it. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in Object Pascal (Delphi) on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-OP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. class procedure ReadValue.Main; var Client: OpcLabs_EasyOpcUA_TLB._EasyUAClient; Value: OleVariant; begin // Instantiate the client object Client := CoEasyUAClient.Create; // Obtain value of a node try Value := Client.ReadValue( //'http://opcua.demo-this.com:51211/UA/SampleServer', //'https://opcua.demo-this.com:51212/UA/SampleServer/', 'opc.tcp://opcua.demo-this.com:51210/UA/SampleServer', 'nsu=http://test.org/UA/Data/ ;i=10853'); except on E: EOleException do begin WriteLn(Format('*** Failure: %s', [E.GetBaseException.Message])); Exit; end; end; // Display results WriteLn('value: ', Value); end;
// This example shows how to read value of a single node, and display it. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in PHP on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-PHP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. // Instantiate the client object $Client = new COM("OpcLabs.EasyOpc.UA.EasyUAClient"); // Perform the operation try { $value = $Client->ReadValue( //"http://opcua.demo-this.com:51211/UA/SampleServer", "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer", "nsu=http://test.org/UA/Data/ ;i=10853"); } catch (com_exception $e) { printf("*** Failure: %s\n", $e->getMessage()); Exit(); } // Display results printf("Value: %s\n", $value);
<!-- This example shows how to read value of a single node, and display it. --> <!----> <!-- Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html .--> <!-- OPC client and subscriber examples in PHP on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-PHP .--> <!-- Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own--> <!-- a commercial license in order to use Online Forums, and we reply to every post.--> <html> <body> <?php // Instantiate the client object $Client = new COM("OpcLabs.EasyOpc.UA.EasyUAClient"); // Perform the operation $value = $Client->ReadValue( //"http://opcua.demo-this.com:51211/UA/SampleServer", "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer", "nsu=http://test.org/UA/Data/ ;i=10853"); // Display results // Read item value and display it printf("Value: %s<br>", $value); ?> </body> </html>
# This example shows how to read value of a single node, and display it. # # The Python for Windows (pywin32) extensions package is needed. Install it using "pip install pypiwin32". # CAUTION: We now recommend using Python.NET package instead. Full set of examples with Python.NET is available! # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . import win32com.client from pywintypes import com_error # Instantiate the client object client = win32com.client.Dispatch('OpcLabs.EasyOpc.UA.EasyUAClient') # Perform the operation try: value = client.ReadValue('opc.tcp://opcua.demo-this.com:51210/UA/SampleServer', 'nsu=http://test.org/UA/Data/ ;i=10853') except com_error as e: print('*** Failure: ' + e.args[2][1] + ': ' + e.args[2][2]) exit() # Display results print('value: ', value)
REM This example shows how to read value of a single node, and display it. REM REM Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . REM OPC client and subscriber examples in Visual Basic on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VB . REM Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own REM a commercial license in order to use Online Forums, and we reply to every post. Public Sub ReadValue_Main_Command_Click() OutputText = "" ' Instantiate the client object Dim Client As New EasyUAClient ' Perform the operation On Error Resume Next Dim value As Variant value = Client.ReadValue("opc.tcp://opcua.demo-this.com:51210/UA/SampleServer", "nsu=http://test.org/UA/Data/ ;i=10853") If Err.Number <> 0 Then OutputText = OutputText & "*** Failure: " & Err.Source & ": " & Err.Description & vbCrLf Exit Sub End If On Error GoTo 0 ' Display results OutputText = OutputText & "value: " & value & vbCrLf End Sub
Rem This example shows how to read value of a single node, and display it. Rem Rem Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . Rem OPC client and subscriber examples in VBScript on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBScript . Rem Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own Rem a commercial license in order to use Online Forums, and we reply to every post. Option Explicit ' Instantiate the client object Dim Client: Set Client = CreateObject("OpcLabs.EasyOpc.UA.EasyUAClient") ' Perform the operation On Error Resume Next Dim value: value = Client.ReadValue("opc.tcp://opcua.demo-this.com:51210/UA/SampleServer", "nsu=http://test.org/UA/Data/ ;i=10853") If Err.Number <> 0 Then WScript.Echo "*** Failure: " & Err.Source & ": " & Err.Description WScript.Quit End If On Error Goto 0 ' Display results WScript.Echo "value: " & value