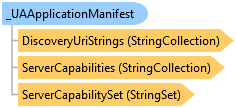
'Declaration
<CLSCompliantAttribute(False)> <ComVisibleAttribute(True)> <GuidAttribute("2C6D6170-BDF2-4932-BDBF-B82E8E8CC6B6")> <InterfaceTypeAttribute(ComInterfaceType.InterfaceIsDual)> Public Interface _UAApplicationManifest
'Usage
Dim instance As _UAApplicationManifest
[CLSCompliant(false)] [ComVisible(true)] [Guid("2C6D6170-BDF2-4932-BDBF-B82E8E8CC6B6")] [InterfaceType(ComInterfaceType.InterfaceIsDual)] public interface _UAApplicationManifest
[CLSCompliant(false)] [ComVisible(true)] [Guid("2C6D6170-BDF2-4932-BDBF-B82E8E8CC6B6")] [InterfaceType(ComInterfaceType.InterfaceIsDual)] public interface class _UAApplicationManifest
This member or type is for use from COM. It is not meant to be used from .NET or Python. Refer to the corresponding .NET member or type instead, if you are developing in .NET or Python.
// This example demonstrates how to set the application name for the application instance certificate. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in Object Pascal (Delphi) on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-OP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. type TClientManagementEventHandlers103 = class procedure OnLogEntry( ASender: TObject; sender: OleVariant; const eventArgs: _LogEntryEventArgs); end; // Event handler for the LogEntry event. // Print the loggable entry containing client certificate parameters. procedure TClientManagementEventHandlers103.OnLogEntry( ASender: TObject; sender: OleVariant; const eventArgs: _LogEntryEventArgs); begin if eventArgs.EventId = 161 then WriteLn(eventArgs.ToString); end; class procedure ApplicationName.Main; var Application: TEasyUAApplication; Client: OpcLabs_EasyOpcUA_TLB._EasyUAClient; ClientManagement: TEasyUAClientManagement; ClientManagementEventHandlers: TClientManagementEventHandlers103; Value: OleVariant; begin // The configuration object allows access to static behavior - here, the // shared LogEntry event. ClientManagement := TEasyUAClientManagement.Create(nil); ClientManagementEventHandlers := TClientManagementEventHandlers103.Create; ClientManagement.OnLogEntry := ClientManagementEventHandlers.OnLogEntry; ClientManagement.Connect; // Obtain the application interface. Application := TEasyUAApplication.Create(nil); try // Set the application name, which determines the subject of the client certificate. // Note that this only works once in each host process. Application.ApplicationParameters.ApplicationManifest.ApplicationName := 'QuickOPC - Delphi example application'; // Do something - invoke an OPC read, to trigger some loggable entries. // If you are doing server development: Instantiate and start the server here, instead of invoking the client. Client := CoEasyUAClient.Create; try Value := Client.ReadValue( //'http://opcua.demo-this.com:51211/UA/SampleServer', //'https://opcua.demo-this.com:51212/UA/SampleServer/', 'opc.tcp://opcua.demo-this.com:51210/UA/SampleServer', 'nsu=http://test.org/UA/Data/ ;i=10853'); except on E: EOleException do begin WriteLn(Format('*** Failure: %s', [E.GetBaseException.Message])); end; end; // The certificate will be located or created in a directory similar to: // C:\ProgramData\OPC Foundation\CertificateStores\MachineDefault\certs // and its subject will be as given by the application name. WriteLn('Processing log entry events for 10 seconds...'); PumpSleep(10*1000); WriteLn('Finished...'); finally FreeAndNil(Application); FreeAndNil(ClientManagement); FreeAndNil(ClientManagementEventHandlers); end; end;
// This example demonstrates how to set the application name for the application instance certificate. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in PHP on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-PHP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. class ClientManagementEvents { // Event handler for the LogEntry event. // Print the loggable entry containing client certificate parameters. function LogEntry($Sender, $E) { if ($E->EventId == 161) printf("%s\n", $E); } } // The management object allows access to static behavior - here, the // shared LogEntry event. $ClientManagement = new COM("OpcLabs.EasyOpc.UA.EasyUAClientManagement"); $ClientManagementEvents = new ClientManagementEvents(); com_event_sink($ClientManagement, $ClientManagementEvents, "DEasyUAClientManagementEvents"); // Obtain the application interface. $Application = new COM("OpcLabs.EasyOpc.UA.Application.EasyUAApplication"); // Set the application name, which determines the subject of the client certificate. // Note that this only works once in each host process. $Application->ApplicationParameters->ApplicationManifest->ApplicationName = "QuickOPC - PHP example application"; // Do something - invoke an OPC read, to trigger some loggable entries. // If you are doing server development: Instantiate and start the server here, instead of invoking the client. $Client = new COM("OpcLabs.EasyOpc.UA.EasyUAClient"); try { $value = $Client->ReadValue( //"http://opcua.demo-this.com:51211/UA/SampleServer", "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer", "nsu=http://test.org/UA/Data/ ;i=10853"); } catch (com_exception $e) { printf("*** Failure: %s\n", $e->getMessage()); } // The certificate will be located or created in a directory similar to: // C:\ProgramData\OPC Foundation\CertificateStores\MachineDefault\certs // and its subject will be as given by the application name. printf("Processing log entry events for 10 seconds..."); $startTime = time(); do { com_message_pump(1000); } while (time() < $startTime + 10); printf("Finished.\n");
REM This example demonstrates how to set the application name for the application instance certificate. REM REM Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . REM OPC client and subscriber examples in Visual Basic on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VB . REM Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own REM a commercial license in order to use Online Forums, and we reply to every post. Private Sub ApplicationName_Main_Command_Click() OutputText = "" ' Obtain the application interface Dim Application As New EasyUAApplication ' Set the application name, which determines the subject of the client certificate. ' Note that this only works once in each host process. Application.ApplicationParameters.ApplicationManifest.applicationName = "QuickOPC - VB6 example application" ' Do something - invoke an OPC read, to trigger some loggable entries. ' If you are doing server development: Instantiate and start the server here, instead of invoking the client. Dim client As New EasyUAClient On Error Resume Next Dim value As Variant value = client.ReadValue("opc.tcp://opcua.demo-this.com:51210/UA/SampleServer", "nsu=http://test.org/UA/Data/ ;i=10853") If Err.Number <> 0 Then OutputText = OutputText & "*** Failure: " & Err.Source & ": " & Err.Description & vbCrLf Exit Sub End If On Error GoTo 0 ' The certificate will be located or created in a directory similar to: ' C:\ProgramData\OPC Foundation\CertificateStores\MachineDefault\certs ' and its subject will be as given by the application name. OutputText = OutputText & "Processing log entry events for 10 seconds..." & vbCrLf Pause 10000 Set Application = Nothing OutputText = OutputText & "Finished..." & vbCrLf End Sub ' Event handler for the LogEntry event. It simply prints out the event. Private Sub ClientManagement1_LogEntry(ByVal sender As Variant, ByVal eventArgs As OpcLabs_BaseLib.LogEntryEventArgs) If eventArgs.eventId = 161 Then OutputText = OutputText & eventArgs & vbCrLf End If End Sub
Rem This example demonstrates how to set the application name for the application instance certificate. Rem Rem Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . Rem OPC client and subscriber examples in VBScript on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBScript . Rem Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own Rem a commercial license in order to use Online Forums, and we reply to every post. Option Explicit ' The management object allows access to static behavior. WScript.Echo "Obtaining the client management object..." Dim ClientManagement: Set ClientManagement = CreateObject("OpcLabs.EasyOpc.UA.EasyUAClientManagement") WScript.ConnectObject ClientManagement, "ClientManagement_" WScript.Echo "Obtaining the application interface..." Dim Application: Set Application = CreateObject("OpcLabs.EasyOpc.UA.Application.EasyUAApplication") ' Set the application name, which determines the subject of the client certificate. ' Note that this only works once in each host process. WScript.Echo "Setting the application name..." Application.ApplicationParameters.ApplicationManifest.ApplicationName = "QuickOPC - VBScript example application" WScript.Echo "Creating a client object..." Dim Client: Set Client = CreateObject("OpcLabs.EasyOpc.UA.EasyUAClient") ' Do something - invoke an OPC read, to trigger some loggable entries. ' If you are doing server development: Instantiate and start the server here, instead of invoking the client. WScript.Echo "Reading a value..." On Error Resume Next Dim value: value = Client.ReadValue("opc.tcp://opcua.demo-this.com:51210/UA/SampleServer", "nsu=http://test.org/UA/Data/ ;i=10853") If Err.Number <> 0 Then WScript.Echo "*** Failure: " & Err.Source & ": " & Err.Description WScript.Quit End If On Error Goto 0 ' The certificate will be located or created in a directory similar to: ' C:\Users\All Users\OPC Foundation\CertificateStores\UA Applications\certs\ ' and its subject will be as given by the application name. WScript.Echo "Processing log entry events for 10 seconds..." WScript.Sleep 10*1000 WScript.Echo "Finished." ' Event handler for the LogEntry event. ' Print the loggable entry containing client certificate parameters. Sub ClientManagement_LogEntry(Sender, e) If e.EventId = 161 Then WScript.Echo e End Sub