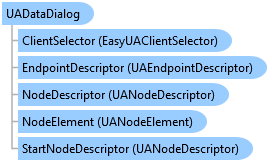
'Declaration
<CLSCompliantAttribute(True)> <ComDefaultInterfaceAttribute(OpcLabs.EasyOpc.UA.Forms.Browsing.ComTypes._UADataDialog)> <ComVisibleAttribute(True)> <GuidAttribute("F444999E-AF8E-4EA8-A425-786B468B1380")> <TypeConverterAttribute(System.ComponentModel.ExpandableObjectConverter)> <DesignerCategoryAttribute("Component")> <SerializableAttribute()> Public NotInheritable Class UADataDialog Inherits UADialog Implements OpcLabs.BaseLib.Forms.ComTypes._FormCommonDialog, OpcLabs.BaseLib.Forms.ComTypes._SizableCommonDialog, OpcLabs.BaseLib.Licensing.ILicensingContextHolder, OpcLabs.EasyOpc.UA.Forms.Browsing.ComTypes._UADataDialog, OpcLabs.EasyOpc.UA.Forms.Browsing.ComTypes._UADialog, System.ComponentModel.IComponent, System.ComponentModel.ISupportInitialize, System.IDisposable
'Usage
Dim instance As UADataDialog
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.EasyOpc.UA.Forms.Browsing.ComTypes._UADataDialog)] [ComVisible(true)] [Guid("F444999E-AF8E-4EA8-A425-786B468B1380")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [DesignerCategory("Component")] [Serializable()] public sealed class UADataDialog : UADialog, OpcLabs.BaseLib.Forms.ComTypes._FormCommonDialog, OpcLabs.BaseLib.Forms.ComTypes._SizableCommonDialog, OpcLabs.BaseLib.Licensing.ILicensingContextHolder, OpcLabs.EasyOpc.UA.Forms.Browsing.ComTypes._UADataDialog, OpcLabs.EasyOpc.UA.Forms.Browsing.ComTypes._UADialog, System.ComponentModel.IComponent, System.ComponentModel.ISupportInitialize, System.IDisposable
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.EasyOpc.UA.Forms.Browsing.ComTypes._UADataDialog)] [ComVisible(true)] [Guid("F444999E-AF8E-4EA8-A425-786B468B1380")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [DesignerCategory("Component")] [Serializable()] public ref class UADataDialog sealed : public UADialog, OpcLabs.BaseLib.Forms.ComTypes._FormCommonDialog, OpcLabs.BaseLib.Forms.ComTypes._SizableCommonDialog, OpcLabs.BaseLib.Licensing.ILicensingContextHolder, OpcLabs.EasyOpc.UA.Forms.Browsing.ComTypes._UADataDialog, OpcLabs.EasyOpc.UA.Forms.Browsing.ComTypes._UADialog, System.ComponentModel.IComponent, System.ComponentModel.ISupportInitialize, System.IDisposable
This is a modal dialog box; therefore, when shown, it blocks the rest of the application until the user has made a choice. When a dialog box is displayed modally, no input (keyboard or mouse click) can occur except to objects on the dialog box. The program must hide or close the dialog box (usually in response to some user action) before input to the calling program can occur.
Icon:
The OPC-UA Data Dialog (UADataDialog class) allows the user to interactively select the OPC data node residing in a specific OPC Unified Architecture server. It also has a different mode (controlled by the UserPickEndpoint property) which allows the user to start the node selection by choosing the host and server endpoint first.
Here is an example of OPC-UA Data dialog in action:
In the default mode (when UserPickEndpoint is false), your code should set the EndpointDescriptor property to specify the OPC Unified Architecture server whose nodes are to be browsed. If you want the user to pick the endpoint, set the UserPickEndpoint property to true; in this case, setting the EndpointDescriptor is not needed on input.
After setting the inputs as needed, your code calls the ShowDialog method. If the result is equal to DialogResult.OK, the user has selected the OPC data node, and information about it can be retrieved from the NodeElement (and also NodeDescriptor) property. If UserPickEndpoint is true, the chosen server endpoint can be retrieved from the EndpointDescriptor property.
The user can browse through the UA Objects, and select from Data Variables or Properties.
When you set the MultiSelect property of the UADataDialog to true, the dialog will allow the user to select any number of OPC-UA nodes. The list below the folders and data nodes (labeled “Selected nodes”) contains the set of nodes that the user has selected in the dialog. The user can freely add nodes to this list, or remove them. The selected set is carried over to next invocation of the dialog, unless you change it from the code.
In the multi-selection mode, the set of nodes selected on input (if any) is in the NodeDescriptors property. On the output, the dialog fills the information about selected nodes into the NodeElements property (and updated the NodeDescriptors property as well).
If UserPickEndpoint is true, each node may come from a different server. In this case, the EndpointDescriptors array contains the server endpoints for each node in NodeDescriptors, with corresponding indices in both arrays. The arrays must have the same length.
If you want to change the parameters of the client object the component uses to perform its OPC operations, you can use the ClientSelector Property.
// This example shows how to let the user browse for an OPC-UA data node (a Data Variable or a Property). // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in C# on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-CSharp . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. using System.Windows.Forms; using OpcLabs.EasyOpc.UA.Forms.Browsing; namespace UAFormsDocExamples._UADataDialog { static partial class ShowDialog { public static void Main1(IWin32Window owner) { var dataDialog = new UADataDialog { EndpointDescriptor = {UrlString = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" }, // or "http://opcua.demo-this.com:51211/UA/SampleServer" (currently not supported) // or "https://opcua.demo-this.com:51212/UA/SampleServer/" UserPickEndpoint = true }; DialogResult dialogResult = dataDialog.ShowDialog(owner); if (dialogResult != DialogResult.OK) return; // Display results MessageBox.Show(owner, $"EndpointDescriptor: {dataDialog.EndpointDescriptor}\r\n" + $"NodeElement: {dataDialog.NodeElement}"); } } }
# This example shows how to let the user browse for an OPC-UA data node # (a Data Variable or a Property). # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . # OPC client and subscriber examples in PowerShell on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-PowerShell . # Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own # a commercial license in order to use Online Forums, and we reply to every post. using namespace OpcLabs.EasyOpc.UA.Forms.Browsing # The path below assumes that the current directory is [ProductDir]/Examples-NET/PowerShell/Windows . Add-Type -Path "../../../Components/Opclabs.QuickOpc/net472/OpcLabs.EasyOpcForms.dll" # Instantiate the dialog object. $dataDialog = New-Object UADataDialog $dataDialog.EndpointDescriptor.UrlString = "http://opcua.demo-this.com:51211/UA/SampleServer" $dataDialog.UserPickEndpoint = $true $dialogResult = $dataDialog.ShowDialog() if ($dialogResult -ne [System.Windows.Forms.DialogResult]::OK) { return } # Display results Write-Host "EndpointDescriptor: $($dataDialog.EndpointDescriptor)" Write-Host "NodeElement: $($dataDialog.NodeElement)"
' This example shows how to let the user browse for an OPC-UA data node (a Data Variable or a Property). ' ' Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . ' OPC client and subscriber examples in VB.NET on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBNET . ' Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own ' a commercial license in order to use Online Forums, and we reply to every post. Imports OpcLabs.EasyOpc.UA.Forms.Browsing Namespace UAFormsDocExamples._UADataDialog Friend Class ShowDialog Shared Sub Main1(owner As IWin32Window) Dim dataDialog = New UADataDialog() With { .EndpointDescriptor = New OpcLabs.EasyOpc.UA.UAEndpointDescriptor With { .UrlString = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" }, .UserPickEndpoint = True } ' or "http://opcua.demo-this.com:51211/UA/SampleServer" (currently not supported) ' or "https://opcua.demo-this.com:51212/UA/SampleServer/" Dim dialogResult As DialogResult = dataDialog.ShowDialog(owner) If dialogResult <> DialogResult.OK Then Return End If ' Display results MessageBox.Show(owner, $"EndpointDescriptor: {dataDialog.EndpointDescriptor}" + Environment.NewLine + $"NodeElement: {dataDialog.NodeElement}") End Sub End Class End Namespace
// This example shows how to let the user browse for an OPC-UA data node (a Data Variable or a Property). // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. #include "stdafx.h" // Includes "QuickOpc.h", and other commonly used files #include "ShowDialog.h" namespace _UADataDialog { void ShowDialog::Main() { // Initialize the COM library CoInitializeEx(NULL, COINIT_MULTITHREADED); { // _UADataDialogPtr DataDialogPtr(__uuidof(UADataDialog)); // DataDialogPtr->EndpointDescriptor->UrlString = //L"http://opcua.demo-this.com:51211/UA/SampleServer"; L"opc.tcp://opcua.demo-this.com:51210/UA/SampleServer"; DataDialogPtr->UserPickEndpoint = true; // DialogResult dialogResult = DataDialogPtr->ShowDialog(NULL); // Display results if (dialogResult == 1/*OK*/) { _tprintf(_T("%d\n"), dialogResult); _tprintf(_T("EndpointDescriptor: %s\n"), (LPCTSTR)CW2CT(DataDialogPtr->EndpointDescriptor->ToString)); _tprintf(_T("NodeElement: %s\n"), (LPCTSTR)CW2CT(DataDialogPtr->NodeElement->ToString)); } } // Release all interface pointers BEFORE calling CoUninitialize() CoUninitialize(); } }
// This example shows how to let the user browse for an OPC-UA data node // (a Data Variable or a Property). // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in Object Pascal (Delphi) on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-OP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. class procedure ShowDialog.Main; var DataDialog: OpcLabs_EasyOpcForms_TLB._UADataDialog; DialogResult: System_Windows_Forms_TLB.DialogResult; begin // Instantiate the dialog object DataDialog := CoUADataDialog.Create; DataDialog.EndpointDescriptor.UrlString := //'http://opcua.demo-this.com:51211/UA/SampleServer'; //'https://opcua.demo-this.com:51212/UA/SampleServer/'; 'opc.tcp://opcua.demo-this.com:51210/UA/SampleServer'; DataDialog.UserPickEndpoint := True; DialogResult := DataDialog.ShowDialog(nil); WriteLn(DialogResult); if DialogResult <> DialogResult_OK then Exit; // Display results WriteLn('EndpointDescriptor: ', DataDialog.EndpointDescriptor.ToString); WriteLn('NodeElement: ', DataDialog.NodeElement.ToString); end;
REM This example shows how to let the user browse for an OPC-UA data node (a Data Variable or a Property). REM REM Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . REM OPC client and subscriber examples in Visual Basic on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VB . REM Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own REM a commercial license in order to use Online Forums, and we reply to every post. Private Sub ShowDialog_Main_Command_Click() OutputText = "" Dim DataDialog As New UADataDialog DataDialog.endpointDescriptor.UrlString = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" DataDialog.UserPickEndpoint = True Dim DialogResult DialogResult = DataDialog.ShowDialog OutputText = OutputText & DialogResult & vbCrLf If DialogResult <> 1 Then ' OK Exit Sub End If ' Display results OutputText = OutputText & "EndpointDescriptor: " & DataDialog.endpointDescriptor & vbCrLf OutputText = OutputText & "NodeElement: " & DataDialog.NodeElement & vbCrLf End Sub
Rem This example shows how to let the user browse for an OPC-UA data node (a Data Variable or a Property). Rem Rem Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . Rem OPC client and subscriber examples in VBScript on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBScript . Rem Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own Rem a commercial license in order to use Online Forums, and we reply to every post. Option Explicit Const DialogResult_OK = 1 Dim DataDialog: Set DataDialog = CreateObject("OpcLabs.EasyOpc.UA.Forms.Browsing.UADataDialog") DataDialog.EndpointDescriptor.UrlString = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" DataDialog.UserPickEndpoint = True Dim dialogResult: dialogResult = DataDialog.ShowDialog WScript.Echo dialogResult If dialogResult <> DialogResult_OK Then WScript.Quit End If ' Display results WScript.Echo "EndpointDescriptor: " & DataDialog.EndpointDescriptor WScript.Echo "NodeElement: " & DataDialog.NodeElement
# This example shows how to let the user browse for an OPC-UA data node (a Data Variable or a Property). # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . # OPC client and subscriber examples in Python on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-Python . # Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own # a commercial license in order to use Online Forums, and we reply to every post. # The QuickOPC package is needed. Install it using "pip install opclabs_quickopc". import opclabs_quickopc # Import .NET namespaces. from System.Windows.Forms import * from OpcLabs.EasyOpc.UA.Forms.Browsing import * dataDialog = UADataDialog() dataDialog.EndpointDescriptor.UrlString = 'opc.tcp://opcua.demo-this.com:51210/UA/SampleServer' # or 'http://opcua.demo-this.com:51211/UA/SampleServer' (currently not supported) # or 'https://opcua.demo-this.com:51212/UA/SampleServer/' dataDialog.UserPickEndpoint = True dialogResult = dataDialog.ShowDialog() print(dialogResult) if dialogResult != DialogResult.OK: exit() # Display results. print('EndpointDescriptor: ', dataDialog.EndpointDescriptor, sep='') print('NodeElement: ', dataDialog.NodeElement, sep='') print('Finished.')
// This example shows how to let the user browse for multiple OPC-UA data nodes (data variables or properties). // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in C# on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-CSharp . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. using System; using System.Linq; using System.Windows.Forms; using OpcLabs.EasyOpc.UA.Forms.Browsing; namespace UAFormsDocExamples._UADataDialog { static partial class ShowDialog { public static void MultiSelect(IWin32Window owner) { var dataDialog = new UADataDialog { EndpointDescriptor = { UrlString = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" }, // or "http://opcua.demo-this.com:51211/UA/SampleServer" (currently not supported) // or "https://opcua.demo-this.com:51212/UA/SampleServer/" MultiSelect = true, UserPickEndpoint = true }; DialogResult dialogResult = dataDialog.ShowDialog(owner); if (dialogResult != DialogResult.OK) return; // Display results MessageBox.Show(owner, String.Join(Environment.NewLine, dataDialog.NodeElements.Select(element => element.ToString()))); } } }
' This example shows how to let the user browse for multiple OPC-UA data nodes (data variables or properties). ' ' Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . ' OPC client and subscriber examples in VB.NET on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBNET . ' Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own ' a commercial license in order to use Online Forums, and we reply to every post. Imports OpcLabs.EasyOpc.UA.Forms.Browsing Namespace UAFormsDocExamples._UADataDialog Partial Friend Class ShowDialog Shared Sub MultiSelect(owner As IWin32Window) Dim dataDialog = New UADataDialog() With { .EndpointDescriptor = New OpcLabs.EasyOpc.UA.UAEndpointDescriptor With { .UrlString = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" }, .MultiSelect = True, .UserPickEndpoint = True } ' or "http://opcua.demo-this.com:51211/UA/SampleServer" (currently not supported) ' or "https://opcua.demo-this.com:51212/UA/SampleServer/" Dim dialogResult As DialogResult = dataDialog.ShowDialog(owner) If dialogResult <> DialogResult.OK Then Return End If ' Display results MessageBox.Show(owner, String.Join(Environment.NewLine, dataDialog.NodeElements.Select(Function(element) element.ToString()))) End Sub End Class End Namespace
# This example shows how to let the user browse for multiple OPC-UA data nodes (data variables or properties). # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . # OPC client and subscriber examples in Python on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-Python . # Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own # a commercial license in order to use Online Forums, and we reply to every post. # The QuickOPC package is needed. Install it using "pip install opclabs_quickopc". import opclabs_quickopc # Import .NET namespaces. from System.Windows.Forms import * from OpcLabs.EasyOpc.UA.Forms.Browsing import * dataDialog = UADataDialog() dataDialog.EndpointDescriptor.UrlString = 'opc.tcp://opcua.demo-this.com:51210/UA/SampleServer' # or 'http://opcua.demo-this.com:51211/UA/SampleServer' (currently not supported) # or 'https://opcua.demo-this.com:51212/UA/SampleServer/' dataDialog.MultiSelect = True dataDialog.UserPickEndpoint = True dialogResult = dataDialog.ShowDialog() print(dialogResult) if dialogResult != DialogResult.OK: exit() # Display results. for nodeElement in dataDialog.NodeElements: print(nodeElement) print() print('Finished.')
System.Object
System.MarshalByRefObject
System.ComponentModel.Component
System.Windows.Forms.CommonDialog
OpcLabs.BaseLib.Forms.ConcreteCommonDialog
OpcLabs.BaseLib.Forms.FormCommonDialog
OpcLabs.BaseLib.Forms.SizableCommonDialog
OpcLabs.EasyOpc.UA.Forms.Browsing.UADialog
OpcLabs.EasyOpc.UA.Forms.Browsing.UADataDialog