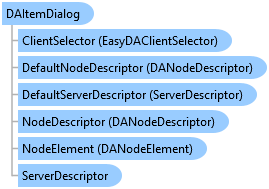
'Declaration
<CLSCompliantAttribute(True)> <ComDefaultInterfaceAttribute(OpcLabs.EasyOpc.DataAccess.Forms.Browsing.ComTypes._DAItemDialog)> <ComVisibleAttribute(True)> <GuidAttribute("211F7E5C-0B3D-4535-AB3D-909D2046B772")> <TypeConverterAttribute(System.ComponentModel.ExpandableObjectConverter)> <DesignerCategoryAttribute("Component")> Public NotInheritable Class DAItemDialog Inherits DADialog Implements OpcLabs.BaseLib.Forms.ComTypes._FormCommonDialog, OpcLabs.BaseLib.Forms.ComTypes._SizableCommonDialog, OpcLabs.BaseLib.Licensing.ILicensingContextHolder, OpcLabs.EasyOpc.DataAccess.Forms.Browsing.ComTypes._DADialog, OpcLabs.EasyOpc.DataAccess.Forms.Browsing.ComTypes._DAItemDialog, System.ComponentModel.IComponent, System.IDisposable
'Usage
Dim instance As DAItemDialog
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.EasyOpc.DataAccess.Forms.Browsing.ComTypes._DAItemDialog)] [ComVisible(true)] [Guid("211F7E5C-0B3D-4535-AB3D-909D2046B772")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [DesignerCategory("Component")] public sealed class DAItemDialog : DADialog, OpcLabs.BaseLib.Forms.ComTypes._FormCommonDialog, OpcLabs.BaseLib.Forms.ComTypes._SizableCommonDialog, OpcLabs.BaseLib.Licensing.ILicensingContextHolder, OpcLabs.EasyOpc.DataAccess.Forms.Browsing.ComTypes._DADialog, OpcLabs.EasyOpc.DataAccess.Forms.Browsing.ComTypes._DAItemDialog, System.ComponentModel.IComponent, System.IDisposable
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.EasyOpc.DataAccess.Forms.Browsing.ComTypes._DAItemDialog)] [ComVisible(true)] [Guid("211F7E5C-0B3D-4535-AB3D-909D2046B772")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [DesignerCategory("Component")] public ref class DAItemDialog sealed : public DADialog, OpcLabs.BaseLib.Forms.ComTypes._FormCommonDialog, OpcLabs.BaseLib.Forms.ComTypes._SizableCommonDialog, OpcLabs.BaseLib.Licensing.ILicensingContextHolder, OpcLabs.EasyOpc.DataAccess.Forms.Browsing.ComTypes._DADialog, OpcLabs.EasyOpc.DataAccess.Forms.Browsing.ComTypes._DAItemDialog, System.ComponentModel.IComponent, System.IDisposable
OpcDAItemDialog is a modal dialog box; therefore, when shown, it blocks the rest of the application until the user has chosen an OPC item. When a dialog box is displayed modally, no input (keyboard or mouse click) can occur except to objects on the dialog box. The program must hide or close the dialog box (usually in response to some user action) before input to the calling program can occur.
The OPC-DA Item Dialog (DAItemDialog class) allows the user to interactively select the OPC item residing in a specific OPC server.
Use the ServerDescriptor property to specify the OPC Data Access server whose items are to be browsed, and call the ShowDialog method. If the result is equal to DialogResult.OK, the user has selected the OPC item, and information about it can be retrieved from the NodeElement property.
The DAItemDialog component retains the filter setting for each node between the invocations of the dialog, making it faster for the user to navigate during the subsequent invocations.
When you set the MultiSelect property of the DAItemDialog to true, the dialog will allow the user to select any number of OPC-DA items. In the multi-select mode, the dialog looks similar to this:
The list below the branches and leaves (labeled “Selected nodes”) contains the set of nodes that the user has selected in the dialog. The user can freely add nodes to this list, or remove them. The selected set is carried over to next invocation of the dialog, unless you change it from the code.
In the multi-selection mode, the set of nodes selected on input (if any) is in the NodeDescriptors property. On the output, the dialog fills the information about selected nodes into the NodeElements property (and updated the NodeDescriptors property as well).
If you want to change the parameters of the client object the component uses to perform its OPC operations, you can use the ClientSelector Property.
// This example shows how to let the user browse for an OPC Data Access item. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in C# on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-CSharp . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. using System.Windows.Forms; using OpcLabs.EasyOpc.DataAccess.Forms.Browsing; namespace FormsDocExamples._DAItemDialog { static class ShowDialog { public static void Main1(IWin32Window owner) { var itemDialog = new DAItemDialog { ServerDescriptor = {ServerClass = "OPCLabs.KitServer.2"} }; DialogResult dialogResult = itemDialog.ShowDialog(owner); if (dialogResult != DialogResult.OK) return; // Display results MessageBox.Show(owner, $"NodeElement: {itemDialog.NodeElement}"); } } }
# This example shows how to let the user browse for an OPC Data Access item. # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . # OPC client and subscriber examples in PowerShell on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-PowerShell . # Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own # a commercial license in order to use Online Forums, and we reply to every post. # The path below assumes that the current directory is [ProductDir]/Examples-NET/PowerShell/Windows . Add-Type -Path "../../../Components/Opclabs.QuickOpc/net472/OpcLabs.EasyOpcForms.dll" $itemDialog = New-Object OpcLabs.EasyOpc.DataAccess.Forms.Browsing.DAItemDialog $itemDialog.ServerDescriptor.ServerClass = "OPCLabs.KitServer.2" $dialogResult = $itemDialog.ShowDialog() if ($dialogResult -ne [System.Windows.Forms.DialogResult]::OK) { return } # Display results Write-Host "NodeElement: $($itemDialog.NodeElement)"
' This example shows how to let the user browse for an OPC Data Access item. ' ' Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . ' OPC client and subscriber examples in VB.NET on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBNET . ' Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own ' a commercial license in order to use Online Forums, and we reply to every post. Imports OpcLabs.EasyOpc Imports OpcLabs.EasyOpc.DataAccess.Forms.Browsing Namespace FormsDocExamples._DAItemDialog Friend Class ShowDialog Shared Sub Main1(owner As IWin32Window) Dim itemDialog = New DAItemDialog() With { .ServerDescriptor = New ServerDescriptor() With { .ServerClass = "OPCLabs.KitServer.2" } } Dim dialogResult As DialogResult = itemDialog.ShowDialog(owner) If dialogResult <> DialogResult.OK Then Return End If ' Display results MessageBox.Show(owner, $"NodeElement: {itemDialog.NodeElement}") End Sub End Class End Namespace
// This example shows how to let the user browse for an OPC Data Access item. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in Object Pascal (Delphi) on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-OP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. class procedure ShowDialog.Main; var ItemDialog: OpcLabs_EasyOpcForms_TLB._DAItemDialog; begin // Instantiate the dialog object ItemDialog := CoDAItemDialog.Create; ItemDialog.ServerDescriptor.ServerClass := 'OPCLabs.KitServer.2'; ItemDialog.ShowDialog(nil); // Display results WriteLn(ItemDialog.NodeElement.ToString); end;
Rem This example shows how to let the user browse for an OPC Data Access item. Rem Rem Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . Rem OPC client and subscriber examples in VBScript on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBScript . Rem Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own Rem a commercial license in order to use Online Forums, and we reply to every post. Option Explicit Const DialogResult_OK = 1 Dim ItemDialog: Set ItemDialog = CreateObject("OpcLabs.EasyOpc.DataAccess.Forms.Browsing.DAItemDialog") ItemDialog.ServerDescriptor.ServerClass = "OPCLabs.KitServer.2" Dim dialogResult: dialogResult = ItemDialog.ShowDialog WScript.Echo dialogResult If dialogResult <> DialogResult_OK Then WScript.Quit End If ' Display results WScript.Echo "NodeElement: " & ItemDialog.NodeElement
# This example shows how to let the user browse for an OPC Data Access item. # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . # OPC client and subscriber examples in Python on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-Python . # Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own # a commercial license in order to use Online Forums, and we reply to every post. # The QuickOPC package is needed. Install it using "pip install opclabs_quickopc". import opclabs_quickopc # Import .NET namespaces. from System.Windows.Forms import * from OpcLabs.EasyOpc.DataAccess.Forms.Browsing import * itemDialog = DAItemDialog() itemDialog.ServerDescriptor.ServerClass = "OPCLabs.KitServer.2" dialogResult = itemDialog.ShowDialog() print(dialogResult) if dialogResult != DialogResult.OK: exit() # Display results. print('NodeElement: ', itemDialog.NodeElement, sep='')
System.Object
System.MarshalByRefObject
System.ComponentModel.Component
System.Windows.Forms.CommonDialog
OpcLabs.BaseLib.Forms.ConcreteCommonDialog
OpcLabs.BaseLib.Forms.FormCommonDialog
OpcLabs.BaseLib.Forms.SizableCommonDialog
OpcLabs.EasyOpc.DataAccess.Forms.Browsing.DADialog
OpcLabs.EasyOpc.DataAccess.Forms.Browsing.DAItemDialog