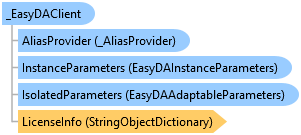
'Declaration
<CLSCompliantAttribute(False)> <ComVisibleAttribute(True)> <GuidAttribute("5D8A341A-863F-41D3-90D5-E88A714CF956")> <InterfaceTypeAttribute(ComInterfaceType.InterfaceIsDual)> Public Interface _EasyDAClient
'Usage
Dim instance As _EasyDAClient
[CLSCompliant(false)] [ComVisible(true)] [Guid("5D8A341A-863F-41D3-90D5-E88A714CF956")] [InterfaceType(ComInterfaceType.InterfaceIsDual)] public interface _EasyDAClient
[CLSCompliant(false)] [ComVisible(true)] [Guid("5D8A341A-863F-41D3-90D5-E88A714CF956")] [InterfaceType(ComInterfaceType.InterfaceIsDual)] public interface class _EasyDAClient
This member or type is for use from COM. It is not meant to be used from .NET or Python. Refer to the corresponding .NET member or type instead, if you are developing in .NET or Python.
// This example shows how to read values of a single item, and display it. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. var Client = new ActiveXObject("OpcLabs.EasyOpc.DataAccess.EasyDAClient"); var value = Client.ReadItemValue("", "OPCLabs.KitServer.2", "Simulation.Random"); WScript.Echo("value: " + value);
// This example shows how to read value of a single item, and display it. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in Object Pascal (Delphi) on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-OP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. class procedure ReadItemValue.Main; var Client: OpcLabs_EasyOpcClassic_TLB._EasyDAClient; Value: OleVariant; begin // Instantiate the client object Client := CoEasyDAClient.Create; try Value := Client.ReadItemValue('', 'OPCLabs.KitServer.2', 'Simulation.Random'); except on E: EOleException do begin WriteLn(Format('*** Failure: %s', [E.GetBaseException.Message])); Exit; end; end; // Display results WriteLn('Value: ', Value); end;
// This example shows how to subscribe to changes of a single item and display the value of the item with each change. // // Some related documentation: http://php.net/manual/en/function.com-event-sink.php . Pay attention to the comment that says // "Be careful how you use this feature; if you are doing something similar to the example below, then it doesn't really make // sense to run it in a web server context.". What they are trying to say is that processing a web request should be // a short-lived code, which does not fit well with the idea of being subscribed to events and received them over longer time. // It is possible to write such code, but it is only useful when processing the request is allowed to take relatively long. Or, // when you are using PHP from command-line, or otherwise - not to serve a web page directly. // // Subscribing to QuickOPC-COM events in the context of PHP Web application, while not imposing the limitations to the request // processing time, has to be "worked around", e.g. using the "event pull" mechanism. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in PHP on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-PHP . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. class DEasyDAClientEvents { function ItemChanged($varSender, $varE) { if ($varE->Succeeded) { print $varE->Vtq->ToString(); print "\n"; } else { printf("*** Failure: %s\n", $varE->ErrorMessageBrief); } } } $Client = new COM("OpcLabs.EasyOpc.DataAccess.EasyDAClient"); $Events = new DEasyDAClientEvents(); com_event_sink($Client, $Events, "DEasyDAClientEvents"); $Client->SubscribeItem("", "OPCLabs.KitServer.2", "Simulation.Random", 1000); print "Processing item changed events for 1 minute...\n"; $startTime = time(); do { com_message_pump(1000); } while (time() < $startTime + 60);
# This example shows how to read value of a single item, and display it. # # The Python for Windows (pywin32) extensions package is needed. Install it using "pip install pypiwin32". # CAUTION: We now recommend using Python.NET package instead. Full set of examples with Python.NET is available! # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . import win32com.client from pywintypes import com_error # Instantiate the client object client = win32com.client.Dispatch('OpcLabs.EasyOpc.DataAccess.EasyDAClient') # Perform the operation try: value = client.ReadItemValue('', 'OPCLabs.KitServer.2', 'Demo.Single') except com_error as e: print('*** Failure: ' + e.args[2][1] + ': ' + e.args[2][2]) exit() # Display results print('value: ', value)
REM This example shows how to read value of a single item, and display it. REM REM Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . REM OPC client and subscriber examples in Visual Basic on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VB . REM Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own REM a commercial license in order to use Online Forums, and we reply to every post. Private Sub ReadItemValue_Main_Command_Click() OutputText = "" ' Instantiate the client object Dim client As New EasyDAClient On Error Resume Next Dim value value = client.ReadItemValue("", "OPCLabs.KitServer.2", "Simulation.Random") If Err.Number <> 0 Then OutputText = OutputText & "*** Failure: " & Err.Source & ": " & Err.Description & vbCrLf Exit Sub End If On Error GoTo 0 ' Display results OutputText = OutputText & "Value: " & value & vbCrLf End Sub
Rem This example shows how to read value of a single item, and display it. Rem Rem Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . Rem OPC client and subscriber examples in VBScript on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBScript . Rem Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own Rem a commercial license in order to use Online Forums, and we reply to every post. Option Explicit Dim Client: Set Client = CreateObject("OpcLabs.EasyOpc.DataAccess.EasyDAClient") On Error Resume Next Dim value: value = Client.ReadItemValue("", "OPCLabs.KitServer.2", "Simulation.Random") If Err.Number <> 0 Then WScript.Echo "*** Failure: " & Err.Source & ": " & Err.Description WScript.Quit End If On Error Goto 0 WScript.Echo "value: " & value