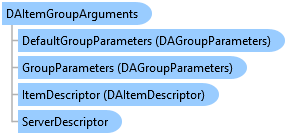
'Declaration
<CLSCompliantAttribute(True)> <ComDefaultInterfaceAttribute(OpcLabs.EasyOpc.DataAccess.OperationModel.ComTypes._DAItemGroupArguments)> <ComVisibleAttribute(True)> <GuidAttribute("9942854B-9730-4367-85F8-FA53300A4216")> <TypeConverterAttribute(System.ComponentModel.ExpandableObjectConverter)> <ValueControlAttribute("OpcLabs.BaseLib.Forms.Common.ObjectSerializationControl, OpcLabs.BaseLibForms, Version=5.81.455.1, Culture=neutral, PublicKeyToken=6faddca41dacb409", DefaultReadWrite=False, Export=True, PageId=10001)> <SerializableAttribute()> Public Class DAItemGroupArguments Inherits DAItemArguments Implements LINQPad.ICustomMemberProvider, OpcLabs.BaseLib.ComTypes._Info, OpcLabs.BaseLib.ComTypes._Object2, OpcLabs.BaseLib.OperationModel.ComTypes._OperationArguments, OpcLabs.EasyOpc.DataAccess.OperationModel.ComTypes._DAItemArguments, OpcLabs.EasyOpc.DataAccess.OperationModel.ComTypes._DAItemGroupArguments, System.ICloneable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
'Usage
Dim instance As DAItemGroupArguments
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.EasyOpc.DataAccess.OperationModel.ComTypes._DAItemGroupArguments)] [ComVisible(true)] [Guid("9942854B-9730-4367-85F8-FA53300A4216")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [ValueControl("OpcLabs.BaseLib.Forms.Common.ObjectSerializationControl, OpcLabs.BaseLibForms, Version=5.81.455.1, Culture=neutral, PublicKeyToken=6faddca41dacb409", DefaultReadWrite=false, Export=true, PageId=10001)] [Serializable()] public class DAItemGroupArguments : DAItemArguments, LINQPad.ICustomMemberProvider, OpcLabs.BaseLib.ComTypes._Info, OpcLabs.BaseLib.ComTypes._Object2, OpcLabs.BaseLib.OperationModel.ComTypes._OperationArguments, OpcLabs.EasyOpc.DataAccess.OperationModel.ComTypes._DAItemArguments, OpcLabs.EasyOpc.DataAccess.OperationModel.ComTypes._DAItemGroupArguments, System.ICloneable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.EasyOpc.DataAccess.OperationModel.ComTypes._DAItemGroupArguments)] [ComVisible(true)] [Guid("9942854B-9730-4367-85F8-FA53300A4216")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [ValueControl("OpcLabs.BaseLib.Forms.Common.ObjectSerializationControl, OpcLabs.BaseLibForms, Version=5.81.455.1, Culture=neutral, PublicKeyToken=6faddca41dacb409", DefaultReadWrite=false, Export=true, PageId=10001)] [Serializable()] public ref class DAItemGroupArguments : public DAItemArguments, LINQPad.ICustomMemberProvider, OpcLabs.BaseLib.ComTypes._Info, OpcLabs.BaseLib.ComTypes._Object2, OpcLabs.BaseLib.OperationModel.ComTypes._OperationArguments, OpcLabs.EasyOpc.DataAccess.OperationModel.ComTypes._DAItemArguments, OpcLabs.EasyOpc.DataAccess.OperationModel.ComTypes._DAItemGroupArguments, System.ICloneable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
Subscription is initiated by calling either SubscribeItem or SubscribeMultipleItems method. For any change in the subscribed item’s value, your application will receive the ItemChanged event notification, described further below. Obviously, you first need to hook up event handler for that event, and in order to prevent event loss, you should do it before subscribing. Alternatively, you can pass a callback method into the SubscribeItem or SubscribeMultipleItems call.
Values of some items may be changing quite frequently, and receiving all changes that are generated is not desirable for performance reasons; there are also physical limitations to the event throughput in the system. Your application needs to specify the requested update rate, which effectively tells the OPC server that you do not need to receive event notifications any faster than that. For OPC items that support it, you can optionally specify a percent deadband; only changes that exceed the deadband will generate an event notification.
In QuickOPC.NET, the requested update rate, percent deadband, and data type are all contained in a DAGroupParameters object.
If you want to subscribe to a specific OPC item, call the SubscribeItem method. You can pass in individual arguments for machine name, server class, ItemID, data type, requested update rate, and an optional percent deadband. Usually, you also pass in a State argument of type Object (in QuickOPC.NET) or VARIANT (in QuickOPC-COM). When the item’s value changes, the State argument is then passed to the ItemChanged event handler in the EasyDAItemChangedEventArgs.Arguments.State property. The SubscribeItem method returns a subscription handle that you can later use to change the subscription parameters, or unsubscribe.
In QuickOPC.NET, you can also pass in a combination of ServerDescriptor, DAItemDescriptor and DAGroupParameters objects, in place of individual arguments.
The State argument is typically used to provide some sort of correlation between objects in your application, and the event notifications. For example, if you are programming an HMI application and you want the event handler to update the control that displays the item’s value, you may want to set the State argument to the control object itself. When the event notification arrives, you simply update the control indicated by the State property of EasyDAItemChangedEventArgs, without having to look it up by ItemId or so. See Use the state instead of handles to identify subscribed entities.
To subscribe to multiple items simultaneously in an efficient manner, call the SubscribeMultipleItems method (instead of multiple SubscribeItem calls in a loop). You receive back an array of integers, which are the subscription handles.
You pass in an array of DAItemGroupArguments objects (each containing information for a single subscription to be made), to the SubscribeMultipleItems method.
Note: It is NOT an error to subscribe to the same item twice (or more times), even with precisely the same parameters. You will receive separate subscription handles, and with regard to your application, this situation will look no different from subscribing to different items. Internally, however, the subscription made to the OPC server will be optimized (merged together) if possible.
You can specify percent deadband when subscribing to an OPC item.
You can also specify the percent deadband when subscribing to multiple OPC items, and the percent deadband can be different for each item.
The example below logs OPC Data Access item changes into an XML file.
The main program:
// This example shows how subscribe to changes of multiple items and display the value of the item with each change. // // Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . // OPC client and subscriber examples in C# on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-CSharp . // Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own // a commercial license in order to use Online Forums, and we reply to every post. using System; using System.Threading; using OpcLabs.EasyOpc.DataAccess; using OpcLabs.EasyOpc.DataAccess.OperationModel; namespace DocExamples.DataAccess._EasyDAClient { partial class SubscribeMultipleItems { public static void Main1() { // Instantiate the client object. using (var client = new EasyDAClient()) { client.ItemChanged += client_Main1_ItemChanged; Console.WriteLine("Subscribing item changes..."); client.SubscribeMultipleItems( new[] { new DAItemGroupArguments("", "OPCLabs.KitServer.2", "Simulation.Random", 1000, null), new DAItemGroupArguments("", "OPCLabs.KitServer.2", "Trends.Ramp (1 min)", 1000, null), new DAItemGroupArguments("", "OPCLabs.KitServer.2", "Trends.Sine (1 min)", 1000, null), new DAItemGroupArguments("", "OPCLabs.KitServer.2", "Simulation.Register_I4", 1000, null) }); Console.WriteLine("Processing item changed events for 1 minute..."); Thread.Sleep(60 * 1000); Console.WriteLine("Unsubscribing item changes..."); } Console.WriteLine("Finished."); } // Item changed event handler static void client_Main1_ItemChanged(object sender, EasyDAItemChangedEventArgs e) { if (e.Succeeded) Console.WriteLine($"{e.Arguments.ItemDescriptor.ItemId}: {e.Vtq}"); else Console.WriteLine($"{e.Arguments.ItemDescriptor.ItemId} *** Failure: {e.ErrorMessageBrief}"); } } }
# This example shows how subscribe to changes of multiple items and display the value of the item with each change. # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . # OPC client and subscriber examples in PowerShell on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-PowerShell . # Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own # a commercial license in order to use Online Forums, and we reply to every post. #requires -Version 5.1 using namespace OpcLabs.EasyOpc.DataAccess using namespace OpcLabs.EasyOpc.DataAccess.OperationModel # The path below assumes that the current directory is [ProductDir]/Examples-NET/PowerShell/Windows . Add-Type -Path "../../../Components/Opclabs.QuickOpc/net472/OpcLabs.EasyOpcClassicCore.dll" Add-Type -Path "../../../Components/Opclabs.QuickOpc/net472/OpcLabs.EasyOpcClassic.dll" Add-Type -Path "../../../Components/Opclabs.QuickOpc/net472/OpcLabs.EasyOpcClassicComponents.dll" # Instantiate the client object. $client = New-Object EasyDAClient # Item changed event handler Register-ObjectEvent -InputObject $client -EventName ItemChanged -Action { if ($EventArgs.Succeeded) { Write-Host "$($EventArgs.Arguments.ItemDescriptor.ItemId): $($EventArgs.Vtq)" } else { Write-Host "$($EventArgs.Arguments.ItemDescriptor.ItemId) *** Failure: $($EventArgs.ErrorMessageBrief)" } } Write-Host "Subscribing item changes..." $handleArray = [IEasyDAClientExtension]::SubscribeMultipleItems($client, @( (New-Object DAItemGroupArguments("", "OPCLabs.KitServer.2", "Simulation.Random", 1000, $null)), (New-Object DAItemGroupArguments("", "OPCLabs.KitServer.2", "Trends.Ramp (1 min)", 1000, $null)), (New-Object DAItemGroupArguments("", "OPCLabs.KitServer.2", "Trends.Sine (1 min)", 1000, $null)), (New-Object DAItemGroupArguments("", "OPCLabs.KitServer.2", "Simulation.Register_I4", 1000, $null)) )) Write-Host "Processing item changed events for 1 minute..." $stopwatch = [System.Diagnostics.Stopwatch]::StartNew() while ($stopwatch.Elapsed.TotalSeconds -lt 30) { Start-Sleep -Seconds 1 } Write-Host "Unsubscribing item changes..." $client.UnsubscribeAllItems() Write-Host "Finished."
' This example shows how subscribe to changes of multiple items and display the value of the item with each change. ' ' Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . ' OPC client and subscriber examples in VB.NET on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-VBNET . ' Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own ' a commercial license in order to use Online Forums, and we reply to every post. Imports System.Threading Imports OpcLabs.EasyOpc.DataAccess Imports OpcLabs.EasyOpc.DataAccess.OperationModel Namespace DataAccess._EasyDAClient Partial Friend Class SubscribeMultipleItems Public Shared Sub Main1() Using client = New EasyDAClient() AddHandler client.ItemChanged, AddressOf client_ItemChanged_Main1 client.SubscribeMultipleItems(New DAItemGroupArguments() { _ New DAItemGroupArguments("", "OPCLabs.KitServer.2", "Simulation.Random", 1000, Nothing), _ New DAItemGroupArguments("", "OPCLabs.KitServer.2", "Trends.Ramp (1 min)", 1000, Nothing), _ New DAItemGroupArguments("", "OPCLabs.KitServer.2", "Trends.Sine (1 min)", 1000, Nothing), _ New DAItemGroupArguments("", "OPCLabs.KitServer.2", "Simulation.Register_I4", 1000, Nothing) _ }) Console.WriteLine("Processing item changed events for 1 minute...") Thread.Sleep(60 * 1000) End Using End Sub ' Item changed event handler Private Shared Sub client_ItemChanged_Main1(ByVal sender As Object, ByVal e As EasyDAItemChangedEventArgs) ' Display the data If e.Succeeded Then Console.WriteLine("{0}: {1}", e.Arguments.ItemDescriptor.ItemId, e.Vtq) Else Console.WriteLine("{0} *** Failure: {1}", e.Arguments.ItemDescriptor.ItemId, e.ErrorMessageBrief) End If End Sub End Class End Namespace
# This example shows how subscribe to changes of multiple items and display the value of the item with each change. # # Find all latest examples here: https://opclabs.doc-that.com/files/onlinedocs/OPCLabs-OpcStudio/Latest/examples.html . # OPC client and subscriber examples in Python on GitHub: https://github.com/OPCLabs/Examples-QuickOPC-Python . # Missing some example? Ask us for it on our Online Forums, https://www.opclabs.com/forum/index ! You do not have to own # a commercial license in order to use Online Forums, and we reply to every post. # The QuickOPC package is needed. Install it using "pip install opclabs_quickopc". import opclabs_quickopc import time # Import .NET namespaces. from OpcLabs.EasyOpc.DataAccess import * from OpcLabs.EasyOpc.DataAccess.OperationModel import * # Item changed event handler. def itemChanged(sender, e): if e.Succeeded: print(e.Arguments.ItemDescriptor.ItemId, ': ', e.Vtq, sep='') else: print(e.Arguments.ItemDescriptor.ItemId, ' *** Failure: ', e.ErrorMessageBrief, sep='') # Instantiate the client object. client = EasyDAClient() client.ItemChanged += itemChanged print('Subscribing item changes...') client.SubscribeMultipleItems([ EasyDAItemSubscriptionArguments('', 'OPCLabs.KitServer.2', 'Simulation.Random', 1000, None), EasyDAItemSubscriptionArguments('', 'OPCLabs.KitServer.2', 'Trends.Ramp (1 min)', 1000, None), EasyDAItemSubscriptionArguments('', 'OPCLabs.KitServer.2', 'Trends.Sine (1 min)', 1000, None), EasyDAItemSubscriptionArguments('', 'OPCLabs.KitServer.2', 'Simulation.Register_I4', 1000, None), ]) print('Processing item change notifications for 1 minute...') time.sleep(60) print('Unsubscribing all items...') client.UnsubscribeAllItems() client.ItemChanged -= itemChanged print('Finished.')
System.Object
OpcLabs.BaseLib.Object2
OpcLabs.BaseLib.Info
OpcLabs.BaseLib.OperationModel.OperationArguments
OpcLabs.EasyOpc.DataAccess.OperationModel.DAItemArguments
OpcLabs.EasyOpc.DataAccess.OperationModel.DAItemGroupArguments
OpcLabs.EasyOpc.DataAccess.OperationModel.EasyDAItemSubscriptionArguments