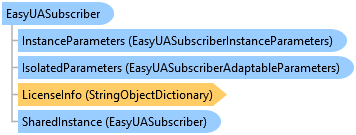
The EasyUASubscriber object is the main object that allows simple access to OPC-UA PubSub publishers.
The core members of this object come from the IEasyUASubscriber interface.
'Declaration
<CLSCompliantAttribute(True)> <ComDefaultInterfaceAttribute(OpcLabs.EasyOpc.UA.PubSub.ComTypes._EasyUASubscriber)> <ComSourceInterfacesAttribute(OpcLabs.EasyOpc.UA.PubSub.ComTypes.DEasyUASubscriberEvents)> <ComVisibleAttribute(True)> <GuidAttribute("EDC1F10E-3FC6-4604-9BC6-4FFF579D271A")> <TypeConverterAttribute(System.ComponentModel.ExpandableObjectConverter)> <DesignerCategoryAttribute("Component")> <SerializableAttribute()> Public NotInheritable Class EasyUASubscriber Inherits EasyUASubscriberCore Implements OpcLabs.BaseLib.ComponentModel.Internal.IComponentNotify, OpcLabs.BaseLib.ILicenseInfoProvider, OpcLabs.BaseLib.IValueEquatable, OpcLabs.BaseLib.Licensing.ILicensingContextHolder, OpcLabs.BaseLib.Widgets.ComTypes._NotifyingWidget, OpcLabs.BaseLib.Widgets.ComTypes._Widget, OpcLabs.BaseLib.Widgets.IWidget, OpcLabs.EasyOpc.UA.PubSub.ComTypes._EasyUASubscriber, IEasyUASubscriber, IEasyUASubscriberSettings, System.ComponentModel.IComponent, System.ICloneable, System.IDisposable, System.IServiceProvider, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
'Usage
Dim instance As EasyUASubscriber
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.EasyOpc.UA.PubSub.ComTypes._EasyUASubscriber)] [ComSourceInterfaces(OpcLabs.EasyOpc.UA.PubSub.ComTypes.DEasyUASubscriberEvents)] [ComVisible(true)] [Guid("EDC1F10E-3FC6-4604-9BC6-4FFF579D271A")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [DesignerCategory("Component")] [Serializable()] public sealed class EasyUASubscriber : EasyUASubscriberCore, OpcLabs.BaseLib.ComponentModel.Internal.IComponentNotify, OpcLabs.BaseLib.ILicenseInfoProvider, OpcLabs.BaseLib.IValueEquatable, OpcLabs.BaseLib.Licensing.ILicensingContextHolder, OpcLabs.BaseLib.Widgets.ComTypes._NotifyingWidget, OpcLabs.BaseLib.Widgets.ComTypes._Widget, OpcLabs.BaseLib.Widgets.IWidget, OpcLabs.EasyOpc.UA.PubSub.ComTypes._EasyUASubscriber, IEasyUASubscriber, IEasyUASubscriberSettings, System.ComponentModel.IComponent, System.ICloneable, System.IDisposable, System.IServiceProvider, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.EasyOpc.UA.PubSub.ComTypes._EasyUASubscriber)] [ComSourceInterfaces(OpcLabs.EasyOpc.UA.PubSub.ComTypes.DEasyUASubscriberEvents)] [ComVisible(true)] [Guid("EDC1F10E-3FC6-4604-9BC6-4FFF579D271A")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [DesignerCategory("Component")] [Serializable()] public ref class EasyUASubscriber sealed : public EasyUASubscriberCore, OpcLabs.BaseLib.ComponentModel.Internal.IComponentNotify, OpcLabs.BaseLib.ILicenseInfoProvider, OpcLabs.BaseLib.IValueEquatable, OpcLabs.BaseLib.Licensing.ILicensingContextHolder, OpcLabs.BaseLib.Widgets.ComTypes._NotifyingWidget, OpcLabs.BaseLib.Widgets.ComTypes._Widget, OpcLabs.BaseLib.Widgets.IWidget, OpcLabs.EasyOpc.UA.PubSub.ComTypes._EasyUASubscriber, IEasyUASubscriber, IEasyUASubscriberSettings, System.ComponentModel.IComponent, System.ICloneable, System.IDisposable, System.IServiceProvider, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
// This example shows how to subscribe to all dataset messages on an OPC-UA PubSub connection with UDP UADP mapping. // // In order to produce network messages for this example, run the UADemoPublisher tool. For documentation, see // http://kb.opclabs.com/UADemoPublisher_Basics . In some cases, you may have to specify the interface name to be used. using System; using System.Collections.Generic; using System.Threading; using OpcLabs.EasyOpc.UA.PubSub; using OpcLabs.EasyOpc.UA.PubSub.OperationModel; namespace UADocExamples.PubSub._EasyUASubscriber { partial class SubscribeDataSet { public static void Main1() { // Define the PubSub connection we will work with. Uses implicit conversion from a string. UAPubSubConnectionDescriptor pubSubConnectionDescriptor = "opc.udp://239.0.0.1"; // In some cases you may have to set the interface (network adapter) name that needs to be used, similarly to // the statement below. Your actual interface name may differ, of course. //pubSubConnectionDescriptor.ResourceAddress.InterfaceName = "Ethernet"; // Instantiate the subscriber object and hook events. var subscriber = new EasyUASubscriber(); subscriber.DataSetMessage += subscriber_DataSetMessage; Console.WriteLine("Subscribing..."); subscriber.SubscribeDataSet(pubSubConnectionDescriptor); Console.WriteLine("Processing dataset message events for 20 seconds..."); Thread.Sleep(20 * 1000); Console.WriteLine("Unsubscribing..."); subscriber.UnsubscribeAllDataSets(); Console.WriteLine("Waiting for 1 second..."); // Unsubscribe operation is asynchronous, messages may still come for a short while. Thread.Sleep(1 * 1000); Console.WriteLine("Finished."); } static void subscriber_DataSetMessage(object sender, EasyUADataSetMessageEventArgs e) { // Display the dataset. if (e.Succeeded) { // An event with null DataSetData just indicates a successful connection. if (!(e.DataSetData is null)) { Console.WriteLine(); Console.WriteLine($"Dataset data: {e.DataSetData}"); foreach (KeyValuePair<string, UADataSetFieldData> pair in e.DataSetData.FieldDataDictionary) Console.WriteLine(pair); } } else { Console.WriteLine(); Console.WriteLine($"*** Failure: {e.ErrorMessageBrief}"); } } // Example output (truncated): // //Subscribing... //Processing dataset message events for 20 seconds... // //Dataset data: Good; Data; publisher="32", writer=1, class=eae79794-1af7-4f96-8401-4096cd1d8908, fields: 4 //[#0, True {System.Boolean} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#1, 7945 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#2, 5246 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#3, 9/30/2019 11:19:14 AM {System.DateTime} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] // //Dataset data: Good; Data; publisher="32", writer=3, class=96976b7b-0db7-46c3-a715-0979884b55ae, fields: 100 //[#0, 45 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#1, 145 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#2, 245 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#3, 345 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#4, 445 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#5, 545 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#6, 645 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#7, 745 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#8, 845 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#9, 945 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#10, 1045 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //... } }
# This example shows how to subscribe to all dataset messages on an OPC-UA PubSub connection with UDP UADP mapping. # # In order to produce network messages for this example, run the UADemoPublisher tool. For documentation, see # http:#kb.opclabs.com/UADemoPublisher_Basics . In some cases, you may have to specify the interface name to be used. #requires -Version 5.1 using namespace OpcLabs.BaseLib.Networking using namespace OpcLabs.EasyOpc.UA using namespace OpcLabs.EasyOpc.UA.PubSub using namespace OpcLabs.EasyOpc.UA.PubSub.OperationModel # The path below assumes that the current directory is [ProductDir]/Examples-NET/PowerShell/Windows . Add-Type -Path "../../../Components/Opclabs.QuickOpc/net472/OpcLabs.EasyOpcUA.dll" Add-Type -Path "../../../Components/Opclabs.QuickOpc/net472/OpcLabs.EasyOpcUAComponents.dll" # Define the PubSub connection we will work with. Uses implicit conversion from a string. [UAPubSubConnectionDescriptor]$pubSubConnectionDescriptor = [ResourceAddress]"opc.udp://239.0.0.1" # In some cases you may have to set the interface (network adapter) name that needs to be used, similarly to # the statement below. Your actual interface name may differ, of course. #$pubSubConnectionDescriptor.ResourceAddress.InterfaceName = "Ethernet" # Instantiate the subscriber object. $subscriber = New-Object EasyUASubscriber # Event notification handler Register-ObjectEvent -InputObject $subscriber -EventName DataSetMessage -Action { # Display the dataset. if ($EventArgs.Succeeded) { # An event with null DataSetData just indicates a successful connection. if ($EventArgs.DataSetData -ne $null) { Write-Host Write-Host "Dataset data: $($EventArgs.DataSetData)" foreach ($pair in $EventArgs.DataSetData.FieldDataDictionary.GetEnumerator()) { Write-Host $pair } } } else { Write-Host Write-Host "*** Failure: $($EventArgs.ErrorMessageBrief)" } } Write-Host "Subscribing..." [IEasyUASubscriberExtension]::SubscribeDataSet($subscriber, $pubSubConnectionDescriptor) Write-Host "Processing dataset message events for 20 seconds..." $stopwatch = [System.Diagnostics.Stopwatch]::StartNew() while ($stopwatch.Elapsed.TotalSeconds -lt 20) { Start-Sleep -Seconds 1 } Write-Host "Unsubscribing..." $subscriber.UnsubscribeAllDataSets() Write-Host "Waiting for 1 second..." # Unsubscribe operation is asynchronous, messages may still come for a short while. Start-Sleep -Seconds 1 Write-Host "Finished." # Example output (truncated): # #Subscribing... #Processing dataset message events for 20 seconds... # #Dataset data: Good; Data; publisher="32", writer=1, class=eae79794-1af7-4f96-8401-4096cd1d8908, fields: 4 #[#0, True {System.Boolean} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#1, 7945 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#2, 5246 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#3, 9/30/2019 11:19:14 AM {System.DateTime} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] # #Dataset data: Good; Data; publisher="32", writer=3, class=96976b7b-0db7-46c3-a715-0979884b55ae, fields: 100 #[#0, 45 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#1, 145 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#2, 245 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#3, 345 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#4, 445 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#5, 545 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#6, 645 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#7, 745 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#8, 845 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#9, 945 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#10, 1045 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #...
# This example shows how to subscribe to all dataset messages on an OPC-UA PubSub connection with UDP UADP mapping. # # In order to produce network messages for this example, run the UADemoPublisher tool. For documentation, see # http://kb.opclabs.com/UADemoPublisher_Basics . In some cases, you may have to specify the interface name to be used. # The QuickOPC package is needed. Install it using "pip install opclabs_quickopc". import opclabs_quickopc import time # Import .NET namespaces. from OpcLabs.EasyOpc.UA.PubSub import * from OpcLabs.EasyOpc.UA.PubSub.OperationModel import * def dataSetMessage(sender, e): # Display the dataset. if e.Succeeded: # An event with null DataSetData just indicates a successful connection. if e.DataSetData is not None: print('') print('Dataset data: ', e.DataSetData, sep='') for pair in e.DataSetData.FieldDataDictionary: print(pair) else: print('') print('*** Failure: ', e.ErrorMessageBrief, sep='') # Define the PubSub connection we will work with. Uses implicit conversion from a string. pubSubConnectionDescriptor = UAPubSubConnectionDescriptor.op_Implicit('opc.udp://239.0.0.1') # In some cases you may have to set the interface (network adapter) name that needs to be used, similarly to # the statement below. Your actual interface name may differ, of course. #pubSubConnectionDescriptor.ResourceAddress.InterfaceName = 'Ethernet' # Instantiate the subscriber object and hook events. subscriber = EasyUASubscriber() subscriber.DataSetMessage += dataSetMessage print('Subscribing...') IEasyUASubscriberExtension.SubscribeDataSet(subscriber, pubSubConnectionDescriptor) print('Processing dataset message events for 20 seconds...') time.sleep(20) print('Unsubscribing...') subscriber.UnsubscribeAllDataSets() print('Waiting for 1 second...') # Unsubscribe operation is asynchronous, messages may still come for a short while. time.sleep(1) subscriber.DataSetMessage -= dataSetMessage print('Finished.') # Example output: # #Subscribing... #Processing dataset message events for 20 seconds... # ##Dataset data: Good; Data; publisher="32", writer=1, class=eae79794-1af7-4f96-8401-4096cd1d8908, fields: 4 #[#0, True {System.Boolean} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#1, 7945 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#2, 5246 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#3, 9/30/2019 11:19:14 AM {System.DateTime} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] # #Dataset data: Good; Data; publisher="32", writer=3, class=96976b7b-0db7-46c3-a715-0979884b55ae, fields: 100 #[#0, 45 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#1, 145 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#2, 245 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#3, 345 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#4, 445 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#5, 545 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#6, 645 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#7, 745 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#8, 845 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#9, 945 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#10, 1045 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #...
' This example shows how to subscribe to all dataset messages on an OPC-UA PubSub connection with UDP UADP mapping. ' ' In order to produce network messages for this example, run the UADemoPublisher tool. For documentation, see ' http://kb.opclabs.com/UADemoPublisher_Basics . In some cases, you may have to specify the interface name to be used. Imports OpcLabs.EasyOpc.UA.PubSub Imports OpcLabs.EasyOpc.UA.PubSub.OperationModel Namespace PubSub._EasyUASubscriber Friend Class SubscribeDataSet Public Shared Sub Main1() ' Define the PubSub connection we will work with. Uses implicit conversion from a string. Dim pubSubConnectionDescriptor As UAPubSubConnectionDescriptor = "opc.udp://239.0.0.1" ' In some cases you may have to set the interface (network adapter) name that needs to be used, similarly to ' the statement below. Your actual interface name may differ, of course. ' pubSubConnectionDescriptor.ResourceAddress.InterfaceName = "Ethernet" ' Instantiate the subscriber object and hook events. Dim subscriber = New EasyUASubscriber() AddHandler subscriber.DataSetMessage, AddressOf subscriber_DataSetMessage Console.WriteLine("Subscribing...") subscriber.SubscribeDataSet(pubSubConnectionDescriptor) Console.WriteLine("Processing dataset message events for 20 seconds...") Threading.Thread.Sleep(20 * 1000) Console.WriteLine("Unsubscribing...") subscriber.UnsubscribeAllDataSets() Console.WriteLine("Waiting for 1 second...") ' Unsubscribe operation is asynchronous, messages may still come for a short while. Threading.Thread.Sleep(1 * 1000) Console.WriteLine("Finished...") End Sub Private Shared Sub subscriber_DataSetMessage(ByVal sender As Object, ByVal e As EasyUADataSetMessageEventArgs) ' Display the dataset. If e.Succeeded Then ' An event with null DataSetData just indicates a successful connection. If e.DataSetData IsNot Nothing Then Console.WriteLine() Console.WriteLine($"Dataset data: {e.DataSetData}") For Each pair As KeyValuePair(Of String, UADataSetFieldData) In e.DataSetData.FieldDataDictionary Console.WriteLine(pair) Next End If Else Console.WriteLine() Console.WriteLine($"*** Failure: {e.ErrorMessageBrief}") End If End Sub End Class ' Example output ' 'Subscribing... 'Processing dataset message events for 20 seconds... ' ''Dataset data: Good; Data; publisher="32", writer=1, class=eae79794-1af7-4f96-8401-4096cd1d8908, fields: 4 '[#0, True {System.Boolean} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#1, 7945 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#2, 5246 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#3, 9/30/2019 11:19:14 AM {System.DateTime} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] ' 'Dataset data: Good; Data; publisher="32", writer=3, class=96976b7b-0db7-46c3-a715-0979884b55ae, fields: 100 '[#0, 45 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#1, 145 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#2, 245 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#3, 345 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#4, 445 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#5, 545 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#6, 645 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#7, 745 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#8, 845 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#9, 945 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#10, 1045 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '... End Namespace
// This example shows how to subscribe to all dataset messages on an OPC-UA PubSub connection with UDP UADP mapping. // // In order to produce network messages for this example, run the UADemoPublisher tool. For documentation, see // http://kb.opclabs.com/UADemoPublisher_Basics . In some cases, you may have to specify the interface name to be used. #include "stdafx.h" // Includes "QuickOpc.h", and other commonly used files #include <atlcom.h> #include "SubscribeDataSet.h" namespace PubSub { namespace _EasyUASubscriber { // CEasyUASubscriberEvents class CEasyUASubscriberEvents : public IDispEventImpl<1, CEasyUASubscriberEvents> { public: BEGIN_SINK_MAP(CEasyUASubscriberEvents) // Event handlers must have the __stdcall calling convention SINK_ENTRY(1, DISPID_EASYUASUBSCRIBEREVENTS_DATASETMESSAGE, &CEasyUASubscriberEvents::DataSetMessage) END_SINK_MAP() public: // The handler for EasyUAClient.DataChangeNotification event STDMETHOD(DataSetMessage)(VARIANT varSender, _EasyUADataSetMessageEventArgs* pEventArgs) { // Display the dataset. if (pEventArgs->Succeeded) { _UADataSetDataPtr DataSetDataPtr = pEventArgs->DataSetData; // An event with null DataSetData just indicates a successful connection. if (DataSetDataPtr != NULL) { _tprintf(_T("\n")); _tprintf(_T("Dataset data: %s\n"), (LPCTSTR)CW2CT(DataSetDataPtr->ToString)); IEnumVARIANTPtr EnumDictionaryEntry2Ptr = DataSetDataPtr->FieldDataDictionary->GetEnumerator(); _variant_t vDictionaryEntry2; while (EnumDictionaryEntry2Ptr->Next(1, &vDictionaryEntry2, NULL) == S_OK) { _DictionaryEntry2Ptr DictionaryEntry2Ptr(vDictionaryEntry2); _tprintf(_T("%s\n"), (LPCTSTR)CW2CT(DictionaryEntry2Ptr->ToString)); vDictionaryEntry2.Clear(); } } } else { _tprintf(_T("\n")); _tprintf(_T("*** Failure: %s\n"), (LPCTSTR)CW2CT(pEventArgs->ErrorMessageBrief)); } return S_OK; } }; void SubscribeDataSet::Main1() { // Initialize the COM library CoInitializeEx(NULL, COINIT_MULTITHREADED); { // Prepare arguments for a subscription to the dataset. _EasyUASubscribeDataSetArgumentsPtr SubscribeDataSetArgumentsPtr(__uuidof(EasyUASubscribeDataSetArguments)); // Define the PubSub connection we will work with. _UAPubSubConnectionDescriptorPtr PubSubConnectionDescriptorPtr = SubscribeDataSetArgumentsPtr->DataSetSubscriptionDescriptor->ConnectionDescriptor; PubSubConnectionDescriptorPtr->ResourceAddress->ResourceDescriptor->UrlString = L"opc.udp://239.0.0.1"; // In some cases you may have to set the interface (network adapter) name that needs to be used, similarly to // the statement below. Your actual interface name may differ, of course. //PubSubConnectionDescriptorPtr->ResourceAddress->InterfaceName = L"Ethernet"; // Instantiate the subscriber object. _EasyUASubscriberPtr SubscriberPtr(__uuidof(EasyUASubscriber)); // Hook events. CEasyUASubscriberEvents* pSubscriberEvents = new CEasyUASubscriberEvents(); AtlGetObjectSourceInterface(SubscriberPtr, &pSubscriberEvents->m_libid, &pSubscriberEvents->m_iid, &pSubscriberEvents->m_wMajorVerNum, &pSubscriberEvents->m_wMinorVerNum); pSubscriberEvents->m_iid = _uuidof(DEasyUASubscriberEvents); pSubscriberEvents->DispEventAdvise(SubscriberPtr, &pSubscriberEvents->m_iid); _tprintf(_T("Subscribing...\n")); _variant_t vSubscribeDataSetArguments(SubscribeDataSetArgumentsPtr.GetInterfacePtr()); SubscriberPtr->SubscribeDataSet(vSubscribeDataSetArguments); _tprintf(_T("Processing dataset message events for 20 seconds...\n")); Sleep(20 * 1000); _tprintf(_T("Unsubscribing...\n")); SubscriberPtr->UnsubscribeAllDataSets(); _tprintf(_T("Waiting for 1 second...\n")); // Unsubscribe operation is asynchronous, messages may still come for a short while. Sleep(1 * 1000); // Unhook events pSubscriberEvents->DispEventUnadvise(SubscriberPtr, &pSubscriberEvents->m_iid); _tprintf(_T("Finished.\n")); } // Release all interface pointers BEFORE calling CoUninitialize() CoUninitialize(); } // Example output: // //Subscribing... //Processing dataset message events for 20 seconds... // ////Dataset data: Good; Data; publisher="32", writer=1, class=eae79794-1af7-4f96-8401-4096cd1d8908, fields: 4 //[#0, True {System.Boolean} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#1, 7945 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#2, 5246 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#3, 9/30/2019 11:19:14 AM {System.DateTime} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] // //Dataset data: Good; Data; publisher="32", writer=3, class=96976b7b-0db7-46c3-a715-0979884b55ae, fields: 100 //[#0, 45 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#1, 145 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#2, 245 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#3, 345 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#4, 445 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#5, 545 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#6, 645 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#7, 745 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#8, 845 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#9, 945 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#10, 1045 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //... } }
// This example shows how to subscribe to all dataset messages on an OPC-UA PubSub connection with UDP UADP mapping. // // In order to produce network messages for this example, run the UADemoPublisher tool. For documentation, see // http://kb.opclabs.com/UADemoPublisher_Basics . In some cases, you may have to specify the interface name to be used. type TSubscriberEventHandlers77 = class procedure OnDataSetMessage( ASender: TObject; sender: OleVariant; const eventArgs: _EasyUADataSetMessageEventArgs); end; class procedure SubscribeDataSet.Main1; var ConnectionDescriptor: _UAPubSubConnectionDescriptor; SubscribeDataSetArguments: _EasyUASubscribeDataSetArguments; Subscriber: TEasyUASubscriber; SubscriberEventHandlers: TSubscriberEventHandlers77; begin // Define the PubSub connection we will work with. SubscribeDataSetArguments := CoEasyUASubscribeDataSetArguments.Create; ConnectionDescriptor := SubscribeDataSetArguments.DataSetSubscriptionDescriptor.ConnectionDescriptor; ConnectionDescriptor.ResourceAddress.ResourceDescriptor.UrlString := 'opc.udp://239.0.0.1'; // In some cases you may have to set the interface (network adapter) name that needs to be used, similarly to // the statement below. Your actual interface name may differ, of course. //ConnectionDescriptor.ResourceAddress.InterfaceName := 'Ethernet'; // Instantiate the subscriber object and hook events. Subscriber := TEasyUASubscriber.Create(nil); SubscriberEventHandlers := TSubscriberEventHandlers77.Create; Subscriber.OnDataSetMessage := SubscriberEventHandlers.OnDataSetMessage; WriteLn('Subscribing...'); Subscriber.SubscribeDataSet(SubscribeDataSetArguments); WriteLn('Processing dataset message for 20 seconds...'); PumpSleep(20*1000); WriteLn('Unsubscribing...'); Subscriber.UnsubscribeAllDataSets; WriteLn('Waiting for 1 second...'); // Unsubscribe operation is asynchronous, messages may still come for a short while. PumpSleep(1*1000); WriteLn('Finished.'); FreeAndNil(Subscriber); FreeAndNil(SubscriberEventHandlers); end; procedure TSubscriberEventHandlers77.OnDataSetMessage( ASender: TObject; sender: OleVariant; const eventArgs: _EasyUADataSetMessageEventArgs); var Count: Cardinal; DictionaryEntry2: _DictionaryEntry2; Element: OleVariant; FieldDataDictionaryEnumerator: IEnumVariant; begin // Display the dataset. if eventArgs.Succeeded then begin // An event with null DataSetData just indicates a successful connection. if eventArgs.DataSetData <> nil then begin WriteLn; WriteLn('Dataset data: ', eventArgs.DataSetData.ToString); FieldDataDictionaryEnumerator := eventArgs.DataSetData.FieldDataDictionary.GetEnumerator; while (FieldDataDictionaryEnumerator.Next(1, Element, Count) = S_OK) do begin DictionaryEntry2 := IUnknown(Element) as _DictionaryEntry2; WriteLn(DictionaryEntry2.ToString); end; end; end else begin WriteLn; WriteLn('*** Failure: ', eventArgs.ErrorMessageBrief); end; end; // Example output: // //Subscribing... //Processing dataset message events for 20 seconds... // ////Dataset data: Good; Data; publisher="32", writer=1, class=eae79794-1af7-4f96-8401-4096cd1d8908, fields: 4 //[#0, True {System.Boolean} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#1, 7945 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#2, 5246 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#3, 9/30/2019 11:19:14 AM {System.DateTime} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] // //Dataset data: Good; Data; publisher="32", writer=3, class=96976b7b-0db7-46c3-a715-0979884b55ae, fields: 100 //[#0, 45 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#1, 145 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#2, 245 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#3, 345 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#4, 445 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#5, 545 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#6, 645 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#7, 745 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#8, 845 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#9, 945 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#10, 1045 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //...
// This example shows how to subscribe to all dataset messages on an OPC-UA PubSub connection with UDP UADP mapping. // // In order to produce network messages for this example, run the UADemoPublisher tool. For documentation, see // http://kb.opclabs.com/UADemoPublisher_Basics . In some cases, you may have to specify the interface name to be used. class SubscriberEvents { function DataSetMessage($Sender, $E) { // Display the dataset. if ($E->Succeeded) { // An event with null DataSetData just indicates a successful connection. printf("\n"); printf("Dataset data: %s\n", $E->DataSetData); foreach ($E->DataSetData->FieldDataDictionary as $Pair) printf("%s\n", $Pair); } else { printf("\n"); printf("*** Failure: %s\n", $E->ErrorMessageBrief); } } } // Define the PubSub connection we will work with. $SubscribeDataSetArguments = new COM("OpcLabs.EasyOpc.UA.PubSub.OperationModel.EasyUASubscribeDataSetArguments"); $ConnectionDescriptor = $SubscribeDataSetArguments->DataSetSubscriptionDescriptor->ConnectionDescriptor; $ConnectionDescriptor->ResourceAddress->ResourceDescriptor->UrlString = "opc.udp://239.0.0.1"; // In some cases you may have to set the interface (network adapter) name that needs to be used, similarly to // the statement below. Your actual interface name may differ, of course. $ConnectionDescriptor->ResourceAddress->InterfaceName = "Ethernet"; // Instantiate the subscriber object and hook events. $Subscriber = new COM("OpcLabs.EasyOpc.UA.PubSub.EasyUASubscriber"); $SubscriberEvents = new SubscriberEvents(); com_event_sink($Subscriber, $SubscriberEvents, "DEasyUASubscriberEvents"); printf("Subscribing...\n"); $Subscriber->SubscribeDataSet($SubscribeDataSetArguments); printf("Processing dataset message events for 20 seconds..."); $startTime = time(); do { com_message_pump(1000); } while (time() < $startTime + 20); printf("Unsubscribing...\n"); $Subscriber->UnsubscribeAllDataSets; printf("Waiting for 1 second..."); // Unsubscribe operation is asynchronous, messages may still come for a short while. $startTime = time(); do { com_message_pump(1000); } while (time() < $startTime + 1); printf("Finished.\n"); // Example output: // //Subscribing... //Processing dataset message events for 20 seconds... // ////Dataset data: Good; Data; publisher="32", writer=1, class=eae79794-1af7-4f96-8401-4096cd1d8908, fields: 4 //[#0, True {System.Boolean} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#1, 7945 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#2, 5246 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#3, 9/30/2019 11:19:14 AM {System.DateTime} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] // //Dataset data: Good; Data; publisher="32", writer=3, class=96976b7b-0db7-46c3-a715-0979884b55ae, fields: 100 //[#0, 45 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#1, 145 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#2, 245 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#3, 345 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#4, 445 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#5, 545 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#6, 645 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#7, 745 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#8, 845 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#9, 945 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //[#10, 1045 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] //...
Rem This example shows how to subscribe to all dataset messages on an OPC-UA PubSub connection with UDP UADP mapping. Rem Rem In order to produce network messages for this example, run the UADemoPublisher tool. For documentation, see Rem http://kb.opclabs.com/UADemoPublisher_Basics . In some cases, you may have to specify the interface name to be used. ' The subscriber object, with events 'Public WithEvents Subscriber2 As EasyUASubscriber Private Sub EasyUASubscriber_SubscribeDataSet_Main1_Command_Click() OutputText = "" ' Define the PubSub connection we will work with. Dim subscribeDataSetArguments As New EasyUASubscribeDataSetArguments Dim ConnectionDescriptor As UAPubSubConnectionDescriptor Set ConnectionDescriptor = subscribeDataSetArguments.dataSetSubscriptionDescriptor.ConnectionDescriptor ConnectionDescriptor.ResourceAddress.ResourceDescriptor.UrlString = "opc.udp://239.0.0.1" ' In some cases you may have to set the interface (network adapter) name that needs to be used, similarly to ' the statement below. Your actual interface name may differ, of course. 'ConnectionDescriptor.ResourceAddress.InterfaceName := 'Ethernet'; ' Instantiate the subscriber object and hook events. Set Subscriber2 = New EasyUASubscriber OutputText = OutputText & "Subscribing..." & vbCrLf Subscriber2.SubscribeDataSet subscribeDataSetArguments OutputText = OutputText & "Processing dataset message for 20 seconds..." & vbCrLf Pause 20000 OutputText = OutputText & "Unsubscribing..." & vbCrLf Subscriber2.UnsubscribeAllDataSets OutputText = OutputText & "Waiting for 1 second..." & vbCrLf ' Unsubscribe operation is asynchronous, messages may still come for a short while. Pause 1000 Set Subscriber2 = Nothing OutputText = OutputText & "Finished." & vbCrLf End Sub Private Sub Subscriber2_DataSetMessage(ByVal sender As Variant, ByVal eventArgs As EasyUADataSetMessageEventArgs) ' Display the dataset If eventArgs.Succeeded Then ' An event with null DataSetData just indicates a successful connection. If Not eventArgs.DataSetData Is Nothing Then OutputText = OutputText & vbCrLf OutputText = OutputText & "Dataset data: " & eventArgs.DataSetData & vbCrLf Dim dictionaryEntry2: For Each dictionaryEntry2 In eventArgs.DataSetData.FieldDataDictionary OutputText = OutputText & dictionaryEntry2 & vbCrLf Next End If Else OutputText = OutputText & vbCrLf OutputText = OutputText & eventArgs.ErrorMessageBrief & vbCrLf End If End Sub ' Example output: ' 'Subscribing... 'Processing dataset message events for 20 seconds... ' 'Dataset data: Good; Data; publisher="32", writer=1, class=eae79794-1af7-4f96-8401-4096cd1d8908, fields: 4 '[#0, True {System.Boolean} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#1, 7945 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#2, 5246 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#3, 9/30/2019 11:19:14 AM {System.DateTime} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] ' 'Dataset data: Good; Data; publisher="32", writer=3, class=96976b7b-0db7-46c3-a715-0979884b55ae, fields: 100 '[#0, 45 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#1, 145 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#2, 245 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#3, 345 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#4, 445 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#5, 545 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#6, 645 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#7, 745 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#8, 845 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#9, 945 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#10, 1045 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '...
Rem This example shows how to subscribe to all dataset messages on an OPC-UA PubSub connection with UDP UADP mapping. Rem Rem In order to produce network messages for this example, run the UADemoPublisher tool. For documentation, see Rem http://kb.opclabs.com/UADemoPublisher_Basics . In some cases, you may have to specify the interface name to be used. Option Explicit ' Define the PubSub connection we will work with. Dim SubscribeDataSetArguments: Set SubscribeDataSetArguments = CreateObject("OpcLabs.EasyOpc.UA.PubSub.OperationModel.EasyUASubscribeDataSetArguments") Dim ConnectionDescriptor: Set ConnectionDescriptor = SubscribeDataSetArguments.DataSetSubscriptionDescriptor.ConnectionDescriptor ConnectionDescriptor.ResourceAddress.ResourceDescriptor.UrlString = "opc.udp://239.0.0.1" ' In some cases you may have to set the interface (network adapter) name that needs to be used, similarly to ' the statement below. Your actual interface name may differ, of course. ' ConnectionDescriptor.ResourceAddress.InterfaceName = "Ethernet" ' Instantiate the subscriber object and hook events. Dim Subscriber: Set Subscriber = CreateObject("OpcLabs.EasyOpc.UA.PubSub.EasyUASubscriber") WScript.ConnectObject Subscriber, "Subscriber_" WScript.Echo "Subscribing..." Subscriber.SubscribeDataSet SubscribeDataSetArguments WScript.Echo "Processing dataset message events for 20 seconds..." WScript.Sleep 20*1000 WScript.Echo "Unsubscribing..." Subscriber.UnsubscribeAllDataSets WScript.Echo "Waiting for 1 second..." ' Unsubscribe operation is asynchronous, messages may still come for a short while. WScript.Sleep 1*1000 WScript.Echo "Finished." Sub Subscriber_DataSetMessage(Sender, e) ' Display the dataset. If e.Succeeded Then ' An event with null DataSetData just indicates a successful connection. If Not (e.DataSetData Is Nothing) Then WScript.Echo WScript.Echo "Dataset data: " & e.DataSetData Dim Pair: For Each Pair in e.DataSetData.FieldDataDictionary WScript.Echo Pair Next End If Else WScript.Echo WScript.Echo "*** Failure: " & e.ErrorMessageBrief End If End Sub ' Example output: ' 'Subscribing... 'Processing dataset message events for 20 seconds... ' ''Dataset data: Good; Data; publisher="32", writer=1, class=eae79794-1af7-4f96-8401-4096cd1d8908, fields: 4 '[#0, True {System.Boolean} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#1, 7945 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#2, 5246 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#3, 9/30/2019 11:19:14 AM {System.DateTime} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] ' 'Dataset data: Good; Data; publisher="32", writer=3, class=96976b7b-0db7-46c3-a715-0979884b55ae, fields: 100 '[#0, 45 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#1, 145 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#2, 245 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#3, 345 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#4, 445 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#5, 545 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#6, 645 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#7, 745 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#8, 845 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#9, 945 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '[#10, 1045 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] '...
# This example shows how to subscribe to all dataset messages on an OPC-UA PubSub connection, and pull events, and # display the incoming datasets. # # In order to produce network messages for this example, run the UADemoPublisher tool. For documentation, see # http://kb.opclabs.com/UADemoPublisher_Basics . In some cases, you may have to specify the interface name to be used. # The QuickOPC package is needed. Install it using "pip install opclabs_quickopc". import opclabs_quickopc import time # Import .NET namespaces. from OpcLabs.EasyOpc.UA.PubSub import * from OpcLabs.EasyOpc.UA.PubSub.OperationModel import * # Define the PubSub connection we will work with. Uses implicit conversion from a string. pubSubConnectionDescriptor = UAPubSubConnectionDescriptor.op_Implicit('opc.udp://239.0.0.1') # In some cases you may have to set the interface (network adapter) name that needs to be used, similarly to # the statement below. Your actual interface name may differ, of course. #pubSubConnectionDescriptor.ResourceAddress.InterfaceName = 'Ethernet' # Instantiate the subscriber object. subscriber = EasyUASubscriber() # In order to use event pull, you must set a non-zero queue capacity upfront. subscriber.PullDataSetMessageQueueCapacity = 1000 print('Subscribing...') IEasyUASubscriberExtension.SubscribeDataSet(subscriber, pubSubConnectionDescriptor) print('Processing dataset message events for 20 seconds...') endTime = time.time() + 20 while time.time() < endTime: eventArgs = IEasyUASubscriberExtension.PullDataSetMessage(subscriber, 2*1000) if eventArgs is not None: # Display the dataset. if eventArgs.Succeeded: # An event with null DataSetData just indicates a successful connection. if eventArgs.DataSetData is not None: print('') print('Dataset data: ', eventArgs.DataSetData, sep='') for pair in eventArgs.DataSetData.FieldDataDictionary: print(pair) else: print('') print('*** Failure: ', eventArgs.ErrorMessageBrief, sep='') print('Unsubscribing...') subscriber.UnsubscribeAllDataSets() print('Waiting for 1 second...') # Unsubscribe operation is asynchronous, messages may still come for a short while. time.sleep(1) print('Finished.') # Example output: # #Subscribing... #Processing dataset message events for 20 seconds... # ##Dataset data: Good; Data; publisher="32", writer=1, class=eae79794-1af7-4f96-8401-4096cd1d8908, fields: 4 #[#0, True {System.Boolean} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#1, 7945 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#2, 5246 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#3, 9/30/2019 11:19:14 AM {System.DateTime} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] # #Dataset data: Good; Data; publisher="32", writer=3, class=96976b7b-0db7-46c3-a715-0979884b55ae, fields: 100 #[#0, 45 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#1, 145 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#2, 245 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#3, 345 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#4, 445 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#5, 545 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#6, 645 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#7, 745 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#8, 845 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#9, 945 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#10, 1045 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #...
# This example shows how to subscribe to all dataset messages on an OPC-UA PubSub connection, and pull events, and display # the incoming datasets. # # In order to produce network messages for this example, run the UADemoPublisher tool. For documentation, see # http://kb.opclabs.com/UADemoPublisher_Basics . In some cases, you may have to specify the interface name to be used. # The Python for Windows (pywin32) extensions package is needed. Install it using "pip install pypiwin32". # CAUTION: We now recommend using Python.NET package instead. Full set of examples with Python.NET is available! import time import win32com.client # Define the PubSub connection we will work with. subscribeDataSetArguments = win32com.client.Dispatch('OpcLabs.EasyOpc.UA.PubSub.OperationModel.EasyUASubscribeDataSetArguments') connectionDescriptor = subscribeDataSetArguments.DataSetSubscriptionDescriptor.ConnectionDescriptor connectionDescriptor.ResourceAddress.ResourceDescriptor.UrlString = 'opc.udp://239.0.0.1' # In some cases you may have to set the interface (network adapter) name that needs to be used, similarly to # the statement below. Your actual interface name may differ, of course. #connectionDescriptor.ResourceAddress.InterfaceName = 'Ethernet' # Instantiate the subscriber object. subscriber = win32com.client.Dispatch('OpcLabs.EasyOpc.UA.PubSub.EasyUASubscriber') # In order to use event pull, you must set a non-zero queue capacity upfront. subscriber.PullDataSetMessageQueueCapacity = 1000 print('Subscribing...') subscriber.SubscribeDataSet(subscribeDataSetArguments) print('Processing dataset message events for 20 seconds...') endTime = time.time() + 20 while time.time() < endTime: eventArgs = subscriber.PullDataSetMessage(2*1000) if eventArgs is not None: # Display the dataset. if eventArgs.Succeeded: # An event with null DataSetData just indicates a successful connection. if eventArgs.DataSetData is not None: print('') print('Dataset data: ', eventArgs.DataSetData) for pair in eventArgs.DataSetData.FieldDataDictionary: print(pair) else: print('') print('*** Failure: ', eventArgs.ErrorMessageBrief) print('Unsubscribing...') subscriber.UnsubscribeAllDataSets print('Waiting for 1 second...') # Unsubscribe operation is asynchronous, messages may still come for a short while. endTime = time.time() + 1 while time.time() < endTime: pass print('Finished.') # Example output: # #Subscribing... #Processing dataset message events for 20 seconds... # ##Dataset data: Good; Data; publisher="32", writer=1, class=eae79794-1af7-4f96-8401-4096cd1d8908, fields: 4 #[#0, True {System.Boolean} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#1, 7945 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#2, 5246 {System.Int32} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#3, 9/30/2019 11:19:14 AM {System.DateTime} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] # #Dataset data: Good; Data; publisher="32", writer=3, class=96976b7b-0db7-46c3-a715-0979884b55ae, fields: 100 #[#0, 45 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#1, 145 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#2, 245 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#3, 345 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#4, 445 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#5, 545 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#6, 645 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#7, 745 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#8, 845 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#9, 945 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #[#10, 1045 {System.Int64} @0001-01-01T00:00:00.000 @@0001-01-01T00:00:00.000; Good] #...
System.Object
System.MarshalByRefObject
System.ComponentModel.Component
OpcLabs.BaseLib.Widgets.Widget
OpcLabs.BaseLib.Widgets.NotifyingWidget
OpcLabs.EasyOpc.UA.PubSub.EasyUASubscriberCore
OpcLabs.EasyOpc.UA.PubSub.EasyUASubscriber