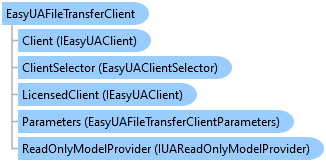
'Declaration
<ComVisibleAttribute(False)> <ExceptionContractVerificationAttribute(True)> <TypeConverterAttribute(System.ComponentModel.ExpandableObjectConverter)> <CLSCompliantAttribute(True)> <DesignerCategoryAttribute("Component")> <SerializableAttribute()> Public NotInheritable Class EasyUAFileTransferClient Inherits EasyUAFileTransferClientCore Implements OpcLabs.BaseLib.Arrangement.IQueryTraits, OpcLabs.BaseLib.IValueEquatable, OpcLabs.BaseLib.Licensing.ILicensingContextHolder, OpcLabs.BaseLib.Widgets.ComTypes._Widget, OpcLabs.BaseLib.Widgets.IWidget, OpcLabs.EasyOpc.UA.ComTypes._EasyUASpecializedClient, IEasyUAFileTransfer, IEasyUAFileTransferClient, OpcLabs.EasyOpc.UA.IEasyUASpecializedClient, System.ComponentModel.IComponent, System.ICloneable, System.IDisposable, System.IServiceProvider, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
'Usage
Dim instance As EasyUAFileTransferClient
[ComVisible(false)] [ExceptionContractVerification(true)] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [CLSCompliant(true)] [DesignerCategory("Component")] [Serializable()] public sealed class EasyUAFileTransferClient : EasyUAFileTransferClientCore, OpcLabs.BaseLib.Arrangement.IQueryTraits, OpcLabs.BaseLib.IValueEquatable, OpcLabs.BaseLib.Licensing.ILicensingContextHolder, OpcLabs.BaseLib.Widgets.ComTypes._Widget, OpcLabs.BaseLib.Widgets.IWidget, OpcLabs.EasyOpc.UA.ComTypes._EasyUASpecializedClient, IEasyUAFileTransfer, IEasyUAFileTransferClient, OpcLabs.EasyOpc.UA.IEasyUASpecializedClient, System.ComponentModel.IComponent, System.ICloneable, System.IDisposable, System.IServiceProvider, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
[ComVisible(false)] [ExceptionContractVerification(true)] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [CLSCompliant(true)] [DesignerCategory("Component")] [Serializable()] public ref class EasyUAFileTransferClient sealed : public EasyUAFileTransferClientCore, OpcLabs.BaseLib.Arrangement.IQueryTraits, OpcLabs.BaseLib.IValueEquatable, OpcLabs.BaseLib.Licensing.ILicensingContextHolder, OpcLabs.BaseLib.Widgets.ComTypes._Widget, OpcLabs.BaseLib.Widgets.IWidget, OpcLabs.EasyOpc.UA.ComTypes._EasyUASpecializedClient, IEasyUAFileTransfer, IEasyUAFileTransferClient, OpcLabs.EasyOpc.UA.IEasyUASpecializedClient, System.ComponentModel.IComponent, System.ICloneable, System.IDisposable, System.IServiceProvider, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
The "file transfer" in the name corresponds to how the related OPC UA specification part is titled. In reality, the functionality covers more than just file transfer - it represents a file system in OPC UA, and provides file access and manipulation operations.
See OPC UA File Transfer Concepts for basic information about what is OPC UA File Transfer and how it works.
See OPC UA File Transfer internals for more information about some internal functionality aspects such as:
// Shows how to read the full contents of an OPC UA file at once, using the file transfer client. // Note: Consider using a higher-level abstraction, OPC UA file provider, instead. using System; using System.IO; using System.Text; using OpcLabs.EasyOpc.UA; using OpcLabs.EasyOpc.UA.FileTransfer; using OpcLabs.EasyOpc.UA.IO.Extensions; namespace UADocExamples.FileTransfer._EasyUAFileTransferClient { class ReadAllBytes { public static void Main1() { // Unified Automation .NET based demo server (UaNETServer/UaServerNET.exe) UAEndpointDescriptor endpointDescriptor = "opc.tcp://localhost:48030"; // A node that represents an instance of OPC UA FileType object. UANodeDescriptor fileNodeDescriptor = "nsu=http://www.unifiedautomation.com/DemoServer/ ;s=Demo.Files.TextFile"; // Instantiate the file transfer client object var fileTransferClient = new EasyUAFileTransferClient(); // Read in all contents from a specified file node. byte[] bytes; try { Console.WriteLine("Reading the whole file..."); bytes = fileTransferClient.ReadAllBytes(endpointDescriptor, fileNodeDescriptor); } // Beware that ReadAllFileBytes throws IOException and not UAException. catch (IOException ioException) { Console.WriteLine("*** Failure: {0}", ioException.GetBaseException().Message); return; } // Display result Console.WriteLine(); // We know that the file contains text, so we convert the received data to a string. If the file contents was // binary, you would process the data according to their format. string text = Encoding.UTF8.GetString(bytes); Console.WriteLine("File content:"); Console.WriteLine(text); Console.WriteLine(); Console.WriteLine("Finished..."); } } }
# Shows how to read the full contents of an OPC UA file at once, using the file transfer client. # Note: Consider using a higher-level abstraction, OPC UA file provider, instead. # The QuickOPC package is needed. Install it using "pip install opclabs_quickopc". import opclabs_quickopc # Import .NET namespaces. from System.Text import * from OpcLabs.EasyOpc.UA import * from OpcLabs.EasyOpc.UA.FileTransfer import * from OpcLabs.EasyOpc.UA.IO.Extensions import * from OpcLabs.EasyOpc.UA.Navigation import * # Unified Automation .NET based demo server (UaNETServer/UaServerNET.exe). endpointDescriptor = UAEndpointDescriptor('opc.tcp://localhost:48030') # A node that represents an instance of OPC UA FileType object. fileNodeDescriptor = UANodeDescriptor('nsu=http://www.unifiedautomation.com/DemoServer/ ;s=Demo.Files.TextFile') # Instantiate the file transfer client object. fileTransferClient = EasyUAFileTransferClient() # Read in all contents from a specified file node. try: print('Reading the whole file...') bytes = IEasyUAFileTransferExtension2.ReadAllBytes(fileTransferClient, endpointDescriptor, UANamedNodeDescriptor(fileNodeDescriptor)) # Beware that ReadAllFileBytes throws IOException and not UAException. except IOException as ioException: print('*** Failure: ' + ioException.GetBaseException().Message) exit() # Display result. print() # We know that the file contains text, so we convert the received data to a string. If the file contents was # binary, you would process the data according to their format. text = Encoding.UTF8.GetString(bytes) print('File content:') print(text) print() print('Finished.')
' Shows how to read the full contents of an OPC UA file at once, using the file transfer client. ' Note: Consider using a higher-level abstraction, OPC UA file provider, instead. Imports System.IO Imports System.Text Imports OpcLabs.EasyOpc.UA Imports OpcLabs.EasyOpc.UA.FileTransfer Imports OpcLabs.EasyOpc.UA.IO.Extensions Namespace FileTransfer._EasyUAFileTransferClient Friend Class ReadAllBytes Public Shared Sub Main1() ' Unified Automation .NET based demo server (UaNETServer/UaServerNET.exe) Dim endpointDescriptor As UAEndpointDescriptor = "opc.tcp://localhost:48030" ' A node that represents an instance of OPC UA FileType object. Dim fileNodeDescriptor As UANodeDescriptor = "nsu=http://www.unifiedautomation.com/DemoServer/ ;s=Demo.Files.TextFile" ' Instantiate the file transfer client object Dim fileTransferClient = New EasyUAFileTransferClient ' Read in all contents from a specified file node. Dim bytes As Byte() Try Console.WriteLine("Reading the whole file...") bytes = fileTransferClient.ReadAllBytes(endpointDescriptor, fileNodeDescriptor) ' Beware that ReadAllFileBytes throws IOException And Not UAException. Catch ioException As IOException Console.WriteLine("*** Failure: {0}", ioException.GetBaseException.Message) Exit Sub End Try ' Display result Console.WriteLine() ' We know that the file contains text, so we convert the received data to a string. If the file contents was ' binary, you would process the data according to their format. Dim text As String = Encoding.UTF8.GetString(bytes) Console.WriteLine("File content:") Console.WriteLine(text) Console.WriteLine() Console.WriteLine("Finished...") End Sub End Class End Namespace
System.Object
System.MarshalByRefObject
System.ComponentModel.Component
OpcLabs.BaseLib.Widgets.Widget
OpcLabs.EasyOpc.UA.EasyUASpecializedClient
OpcLabs.EasyOpc.UA.FileTransfer.EasyUAFileTransferClientCore
OpcLabs.EasyOpc.UA.FileTransfer.EasyUAFileTransferClient