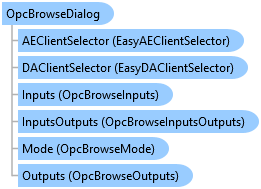
'Declaration
<CLSCompliantAttribute(True)> <ComDefaultInterfaceAttribute(OpcLabs.EasyOpc.Forms.Browsing.ComTypes._OpcBrowseDialog)> <ComVisibleAttribute(True)> <GuidAttribute("DF6649D4-A24A-4AA0-AA62-7FAC59FE2E99")> <TypeConverterAttribute(System.ComponentModel.ExpandableObjectConverter)> <DesignerCategoryAttribute("Component")> Public NotInheritable Class OpcBrowseDialog Inherits OpcLabs.BaseLib.Forms.Browsing.Generalized.BrowseDialog Implements OpcLabs.BaseLib.Forms.ComTypes._FormCommonDialog, OpcLabs.BaseLib.Forms.ComTypes._SizableCommonDialog, OpcLabs.BaseLib.Licensing.ILicensingContextHolder, OpcLabs.EasyOpc.Forms.Browsing.ComTypes._OpcBrowseDialog, System.ComponentModel.IComponent, System.IDisposable
'Usage
Dim instance As OpcBrowseDialog
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.EasyOpc.Forms.Browsing.ComTypes._OpcBrowseDialog)] [ComVisible(true)] [Guid("DF6649D4-A24A-4AA0-AA62-7FAC59FE2E99")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [DesignerCategory("Component")] public sealed class OpcBrowseDialog : OpcLabs.BaseLib.Forms.Browsing.Generalized.BrowseDialog, OpcLabs.BaseLib.Forms.ComTypes._FormCommonDialog, OpcLabs.BaseLib.Forms.ComTypes._SizableCommonDialog, OpcLabs.BaseLib.Licensing.ILicensingContextHolder, OpcLabs.EasyOpc.Forms.Browsing.ComTypes._OpcBrowseDialog, System.ComponentModel.IComponent, System.IDisposable
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.EasyOpc.Forms.Browsing.ComTypes._OpcBrowseDialog)] [ComVisible(true)] [Guid("DF6649D4-A24A-4AA0-AA62-7FAC59FE2E99")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [DesignerCategory("Component")] public ref class OpcBrowseDialog sealed : public OpcLabs.BaseLib.Forms.Browsing.Generalized.BrowseDialog, OpcLabs.BaseLib.Forms.ComTypes._FormCommonDialog, OpcLabs.BaseLib.Forms.ComTypes._SizableCommonDialog, OpcLabs.BaseLib.Licensing.ILicensingContextHolder, OpcLabs.EasyOpc.Forms.Browsing.ComTypes._OpcBrowseDialog, System.ComponentModel.IComponent, System.IDisposable
This is a modal dialog box; therefore, when shown, it blocks the rest of the application until the user has made a selection. When a dialog box is displayed modally, no input (keyboard or mouse click) can occur except to objects on the dialog box. The program must hide or close the dialog box (usually in response to some user action) before input to the calling program can occur.
Icon:
With OpcBrowseDialog, your application can integrate a dialog with various OPC nodes from which the user can select. This dialog can be configured to serve many different purposes.
Here is an example of the generic OPC browsing dialog in action:
The way the dialog operates is controlled by two main properties:
Values of these properties can be selected from the OpcElementType enumeration, which has members for various types of elements that you encounter when working with OPC.
The following chart shows a hierarchy of element types that you can choose from:
For example, let’ say that you set Mode.AnchorElementType to Server, and Mode.SelectElementType to DAProperty. This will cause the dialog to allow the user to browse for an OPC Item (node) on the server you specify, and then for an OPC property on that item.
In this case, before you run the dialog, you need to provide it with values for the InputOutputs.CurrentNodeDescriptor.ServerDescriptor.Location and InputOutputs.CurrentNodeDescriptor.ServerDescriptor.ServerClass properties, because those define your “anchor” element (Server) that the user cannot change. The dialog will only allow the user to finalize it (besides cancelling) after an OPC property is fully selected, because that is your Mode.SelectElementType. After the dialog is successfully finalized, the information about the user’s choice will be available in the Outputs.CurrentNodeElement.DANodeElement and Outputs.CurrentNodeElement.DAPropertyElement properties.
Note that in addition to the “minimal” scenario described above, you can also pre-set the initial node or property, using the InputOutputs.CurrentNodeDescriptor.DANodeDescriptor or InputOutputs.CurrentNodeDescriptor.DAPropertyDescriptor.PropertyId properties, and after the selection is made, these properties will be updated to the new selection as well. This way, if you run the dialog again with the same value, the initial selection will be where the user has left it the last time the dialog was run.
Obviously, the chosen Mode.SelectElementType must be a child or indirect ancestor of chosen Mode.AnchorElementType in the hierarchy. For example, it would be an error to set Mode.AnchorElementType to AECategory and Mode.SelectElementType to DAProperty.
It is also possible to configure the dialog for a multi-selection. In this mode, the user can select zero, one, or more nodes. In order to enable the multi-select mode, set the Mode.MultiSelect property to true. In the multi-select mode, the initial set of the selected nodes (when the dialog is first displayed to the user) is given by the contents of the InputOutputs.SelectionDescriptors collection. When the user makes the selection and accepts it by closing the dialog, this collection is updated, and also, all information about the selected nodes is placed to the Outputs.SelectionElements collection.
There are also ways to control some finer aspects of the dialog. For example, the Mode.ShowListBranches property (defaults to true) controls whether the branches of the tree are also displayed in the list view.
If you want to change the parameters of the client objects the component uses to perform its OPC operations, you can use the DAClientSelector Property or the AEClientSelector Property.
// This example shows how to let the user browse for an OPC Data Access node in a dialog. using System.Windows.Forms; using OpcLabs.EasyOpc.Forms.Browsing; namespace FormsDocExamples._OpcBrowseDialog { static class ShowDialog { public static void Main1(IWin32Window owner) { var browseDialog = new OpcBrowseDialog(); DialogResult dialogResult = browseDialog.ShowDialog(owner); if (dialogResult != DialogResult.OK) return; // Display results MessageBox.Show(owner, browseDialog.Outputs.CurrentNodeElement.DANodeElement); } } }
# This example shows how to let the user browse for an OPC Data Access node in a dialog. # The path below assumes that the current directory is [ProductDir]/Examples-NET/PowerShell/Windows . Add-Type -Path "../../../Components/Opclabs.QuickOpc/net472/OpcLabs.EasyOpcForms.dll" $browseDialog = New-Object OpcLabs.EasyOpc.Forms.Browsing.OpcBrowseDialog $dialogResult = $browseDialog.ShowDialog() if ($dialogResult -ne [System.Windows.Forms.DialogResult]::OK) { return } # Display results Write-Host $browseDialog.Outputs.CurrentNodeElement.DANodeElement
// This example shows how to let the user browse for an OPC Data Access node. class procedure ShowDialog.Main; var BrowseDialog: OpcLabs_EasyOpcForms_TLB._OpcBrowseDialog; begin // Instantiate the dialog object BrowseDialog := CoOpcBrowseDialog.Create; BrowseDialog.ShowDialog(nil); // Display results WriteLn(BrowseDialog.Outputs.CurrentNodeElement.DANodeElement.ToString); end;
Rem This example shows how to let the user browse for an OPC Data Access node. Private Sub ShowDialog_Main_Command_Click() OutputText = "" ' Instantiate the dialog object Dim browseDialog As New OpcBrowseDialog Dim dialogResult dialogResult = browseDialog.ShowDialog OutputText = OutputText & dialogResult & vbCrLf If dialogResult <> 1 Then ' OK Exit Sub End If ' Display results OutputText = OutputText & browseDialog.Outputs.CurrentNodeElement.DANodeElement & vbCrLf End Sub
Rem This example shows how to let the user browse for an OPC Data Access node. Option Explicit Const DialogResult_OK = 1 Dim BrowseDialog: Set BrowseDialog = CreateObject("OpcLabs.EasyOpc.Forms.Browsing.OpcBrowseDialog") Dim dialogResult: dialogResult = BrowseDialog.ShowDialog WScript.Echo dialogResult If dialogResult <> DialogResult_OK Then WScript.Quit End If ' Display results WScript.Echo BrowseDialog.Outputs.CurrentNodeElement.DANodeElement
System.Object
System.MarshalByRefObject
System.ComponentModel.Component
System.Windows.Forms.CommonDialog
OpcLabs.BaseLib.Forms.ConcreteCommonDialog
OpcLabs.BaseLib.Forms.FormCommonDialog
OpcLabs.BaseLib.Forms.SizableCommonDialog
OpcLabs.BaseLib.Forms.Browsing.Generalized.BrowseDialog
OpcLabs.EasyOpc.Forms.Browsing.OpcBrowseDialog