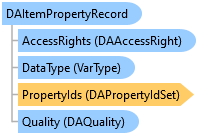
'Declaration
<ComVisibleAttribute(False)> <SerializableAttribute()> Public Class DAItemPropertyRecord
'Usage
Dim instance As DAItemPropertyRecord
[ComVisible(false)] [Serializable()] public class DAItemPropertyRecord
[ComVisible(false)] [Serializable()] public ref class DAItemPropertyRecord
The GetPropertyValueDictionary method allows you to obtain a dictionary of property values for a given OPC item, where a key to the dictionary is the property Id. You can pass in a set of property Ids that you are interested in, or have the method obtain all well-known OPC properties. You can then easily extract the value of any property by looking it up in a dictionary (as opposed to having to numerically index into an array, as with the base GetMultiplePropertyValues method).
The GetItemPropertyRecord method allows you to obtain a structure filled in with property values for a given OPC item. It can retrieve all well-known properties at once, or you can pass in a set of property Ids that you are interested in. You can then simply use the properties in the resulting DAItemPropertyRecord structure, without further looking up the values in any way.
The static DAPropertyIDSet class gives you an easy way to provide pre-defined sets of properties to the above methods. There are well-known sets such as the Basic property set, Extension set, or Alarms and Events property set. It also allows you to combine the property sets together (a union operation), with the Add method or the ‘+’ operator.
System.Object
OpcLabs.EasyOpc.DataAccess.Extensions.DAItemPropertyRecord
Target Platforms: .NET Framework: Windows 10 (selected versions), Windows 11 (selected versions), Windows Server 2012, Windows Server 2016; .NET Core, .NET 5, .NET 6: Linux, macOS, Microsoft Windows