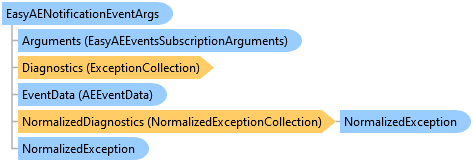
'Declaration
<CLSCompliantAttribute(True)> <ComDefaultInterfaceAttribute(OpcLabs.EasyOpc.AlarmsAndEvents.OperationModel.ComTypes._EasyAENotificationEventArgs)> <ComVisibleAttribute(True)> <GuidAttribute("BC877226-8A44-4389-B95E-3DD44950FEF5")> <TypeConverterAttribute(System.ComponentModel.ExpandableObjectConverter)> <SerializableAttribute()> Public NotInheritable Class EasyAENotificationEventArgs Inherits OpcLabs.BaseLib.OperationModel.OperationEventArgs Implements OpcLabs.BaseLib.OperationModel.ComTypes._OperationEventArgs, OpcLabs.EasyOpc.AlarmsAndEvents.OperationModel.ComTypes._EasyAENotificationEventArgs, System.ICloneable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
'Usage
Dim instance As EasyAENotificationEventArgs
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.EasyOpc.AlarmsAndEvents.OperationModel.ComTypes._EasyAENotificationEventArgs)] [ComVisible(true)] [Guid("BC877226-8A44-4389-B95E-3DD44950FEF5")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [Serializable()] public sealed class EasyAENotificationEventArgs : OpcLabs.BaseLib.OperationModel.OperationEventArgs, OpcLabs.BaseLib.OperationModel.ComTypes._OperationEventArgs, OpcLabs.EasyOpc.AlarmsAndEvents.OperationModel.ComTypes._EasyAENotificationEventArgs, System.ICloneable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.EasyOpc.AlarmsAndEvents.OperationModel.ComTypes._EasyAENotificationEventArgs)] [ComVisible(true)] [Guid("BC877226-8A44-4389-B95E-3DD44950FEF5")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [Serializable()] public ref class EasyAENotificationEventArgs sealed : public OpcLabs.BaseLib.OperationModel.OperationEventArgs, OpcLabs.BaseLib.OperationModel.ComTypes._OperationEventArgs, OpcLabs.EasyOpc.AlarmsAndEvents.OperationModel.ComTypes._EasyAENotificationEventArgs, System.ICloneable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
When an OPC Alarms and Events server generates an event, the EasyAEClient object generates a Notification event. For subscription mechanism to be useful, you should hook one or more event handlers to this event.
To be more precise, the Notification event is actually generated in other cases, too - if there is any significant occurrence related to the event subscription. This can be for three reasons:
The notification for the Notification event contains an EasyAENotificationEventArgs argument. You will find all kind of relevant data in this object. Some properties in this object contain valid information under all circumstances. These properties are e.g. Arguments (including Arguments.State). Other properties, such as EventData, contain null references when there is no associated information for them. When the EventData property is not a null reference, it contains an AEEventData object describing the detail of the actual OPC event received from the OPC Alarms and Events server.
Before further processing, your code should always inspect the value of Exception property of the event arguments. If this property is not a null reference, there has been an error related to the event subscription, the Exception property contains information about the problem, and the EventData property does not contain a valid object.
If the Exception property is a null reference, the notification may be informing you about the fact that a “forced” refresh is complete (in this case, the RefreshComplete property is 'true'), or that an event subscription has been successfully connected or re-connected (in this case, the EventData property is a null reference). If none of the previous applies, the EventData property contains a valid AEEventData object with details about the actual OPC event generated by the OPC server.
Pseudo-code for the full Notification event handler may look similar to this:
if notificationEventArgs.Exception is not null then
An error occurred and the subscription is disconnected, handle it (or ignore)
else if notificationEventArgs.RefreshComplete then
A “refresh” is complete; handle it (only needed if you are invoking a refresh explicitly)
else if notificationEventArgs.EventData is null then
Subscription has been successfully connected or re-connected, handle it (or ignore)
else
Handle the OPC event, details are in notificationEventArgs.EventData. You may use notificationEventArgs.Refresh flag for distinguishing refreshes from original notifications.
The Notification event handler is called on a thread determined by the EasyAEClient component. For details, please refer to “Multithreading and Synchronization” chapter under “Advanced Topics”.
System.Object
System.EventArgs
OpcLabs.BaseLib.OperationModel.OperationEventArgs
OpcLabs.EasyOpc.AlarmsAndEvents.OperationModel.EasyAENotificationEventArgs
Target Platforms: .NET Framework: Windows 10 (selected versions), Windows 11 (selected versions), Windows Server 2012, Windows Server 2016; .NET Core, .NET 5, .NET 6: Linux, macOS, Microsoft Windows