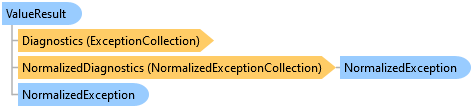
'Declaration
<CLSCompliantAttribute(True)> <ComDefaultInterfaceAttribute(OpcLabs.BaseLib.OperationModel.ComTypes._ValueResult)> <ComVisibleAttribute(True)> <GuidAttribute("B7F6D964-ABA2-4E36-9D96-0E3096F615D7")> <TypeConverterAttribute(System.ComponentModel.ExpandableObjectConverter)> <ValueControlAttribute("OpcLabs.BaseLib.Forms.Common.ObjectSerializationControl, OpcLabs.BaseLibForms, Version=5.72.465.1, Culture=neutral, PublicKeyToken=6faddca41dacb409", DefaultReadWrite=False, Export=True, PageId=10001)> <SerializableAttribute()> Public Class ValueResult Inherits OperationResult Implements OpcLabs.BaseLib.ComTypes._Info, OpcLabs.BaseLib.ComTypes._Object2, OpcLabs.BaseLib.OperationModel.ComTypes._OperationResult, OpcLabs.BaseLib.OperationModel.ComTypes._ValueResult, System.Collections.IStructuralEquatable, System.ICloneable, System.IFormattable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
'Usage
Dim instance As ValueResult
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.BaseLib.OperationModel.ComTypes._ValueResult)] [ComVisible(true)] [Guid("B7F6D964-ABA2-4E36-9D96-0E3096F615D7")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [ValueControl("OpcLabs.BaseLib.Forms.Common.ObjectSerializationControl, OpcLabs.BaseLibForms, Version=5.72.465.1, Culture=neutral, PublicKeyToken=6faddca41dacb409", DefaultReadWrite=false, Export=true, PageId=10001)] [Serializable()] public class ValueResult : OperationResult, OpcLabs.BaseLib.ComTypes._Info, OpcLabs.BaseLib.ComTypes._Object2, OpcLabs.BaseLib.OperationModel.ComTypes._OperationResult, OpcLabs.BaseLib.OperationModel.ComTypes._ValueResult, System.Collections.IStructuralEquatable, System.ICloneable, System.IFormattable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
[CLSCompliant(true)] [ComDefaultInterface(OpcLabs.BaseLib.OperationModel.ComTypes._ValueResult)] [ComVisible(true)] [Guid("B7F6D964-ABA2-4E36-9D96-0E3096F615D7")] [TypeConverter(System.ComponentModel.ExpandableObjectConverter)] [ValueControl("OpcLabs.BaseLib.Forms.Common.ObjectSerializationControl, OpcLabs.BaseLibForms, Version=5.72.465.1, Culture=neutral, PublicKeyToken=6faddca41dacb409", DefaultReadWrite=false, Export=true, PageId=10001)] [Serializable()] public ref class ValueResult : public OperationResult, OpcLabs.BaseLib.ComTypes._Info, OpcLabs.BaseLib.ComTypes._Object2, OpcLabs.BaseLib.OperationModel.ComTypes._OperationResult, OpcLabs.BaseLib.OperationModel.ComTypes._ValueResult, System.Collections.IStructuralEquatable, System.ICloneable, System.IFormattable, System.Runtime.Serialization.ISerializable, System.Xml.Serialization.IXmlSerializable
The result is successful if the Exception is a null reference. Otherwise, this property contains information about the reason of the failure.
// This example shows how to read the Value attributes of 3 different nodes at once. Using the same method, it is also possible // to read multiple attributes of the same node. using System; using OpcLabs.BaseLib.OperationModel; using OpcLabs.EasyOpc.UA; using OpcLabs.EasyOpc.UA.OperationModel; namespace UADocExamples._EasyUAClient { partial class ReadMultipleValues { public static void Main1() { UAEndpointDescriptor endpointDescriptor = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer"; // or "http://opcua.demo-this.com:51211/UA/SampleServer" (currently not supported) // or "https://opcua.demo-this.com:51212/UA/SampleServer/" // Instantiate the client object. var client = new EasyUAClient(); // Obtain values. By default, the Value attributes of the nodes will be read. ValueResult[] valueResultArray = client.ReadMultipleValues(new[] { new UAReadArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10845"), new UAReadArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10853"), new UAReadArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10855") }); // Display results. foreach (ValueResult valueResult in valueResultArray) { if (valueResult.Succeeded) Console.WriteLine($"Value: {valueResult.Value}"); else Console.WriteLine($"*** Failure: {valueResult.ErrorMessageBrief}"); } // Example output: // //Value: 8 //Value: -8.06803E+21 //Value: Strawberry Pig Banana Snake Mango Purple Grape Monkey Purple? Blueberry Lemon^ } } }
# This example shows how to read the Value attributes of 3 different nodes at once. Using the same method, it is also possible # to read multiple attributes of the same node. # The QuickOPC package is needed. Install it using "pip install opclabs_quickopc". import opclabs_quickopc import time # Import .NET namespaces. from OpcLabs.EasyOpc.UA import * from OpcLabs.EasyOpc.UA.OperationModel import * endpointDescriptor = UAEndpointDescriptor('opc.tcp://opcua.demo-this.com:51210/UA/SampleServer') # or 'http://opcua.demo-this.com:51211/UA/SampleServer' (currently not supported) # or 'https://opcua.demo-this.com:51212/UA/SampleServer/' # Instantiate the client object. client = EasyUAClient() # Obtain values. By default, the Value attributes of the nodes will be read. valueResultArray = IEasyUAClientExtension.ReadMultipleValues(client, [ UAReadArguments(endpointDescriptor, UANodeDescriptor('nsu=http://test.org/UA/Data/ ;i=10845')), UAReadArguments(endpointDescriptor, UANodeDescriptor('nsu=http://test.org/UA/Data/ ;i=10853')), UAReadArguments(endpointDescriptor, UANodeDescriptor('nsu=http://test.org/UA/Data/ ;i=10855')), ]) # Display results. for valueResult in valueResultArray: if valueResult.Succeeded: print('Value: ', valueResult.Value, sep='') else: print('*** Failure: ', valueResult.ErrorMessageBrief, sep='') print() print('Finished.')
# This example shows how to read the Value attributes of 3 different nodes at once. Using the same method, it is also possible # to read multiple attributes of the same node. #requires -Version 5.1 using namespace OpcLabs.EasyOpc.UA using namespace OpcLabs.EasyOpc.UA.OperationModel # The path below assumes that the current directory is [ProductDir]/Examples-NET/PowerShell/Windows . Add-Type -Path "../../../Components/Opclabs.QuickOpc/net472/OpcLabs.EasyOpcUA.dll" Add-Type -Path "../../../Components/Opclabs.QuickOpc/net472/OpcLabs.EasyOpcUAComponents.dll" [UAEndpointDescriptor]$endpointDescriptor = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" # or "http://opcua.demo-this.com:51211/UA/SampleServer" (currently not supported) # or "https://opcua.demo-this.com:51212/UA/SampleServer/" # Instantiate the client object. $client = New-Object EasyUAClient # Obtain values. By default, the Value attributes of the nodes will be read. $valueResultArray = $client.ReadMultipleValues([UAReadArguments[]]@( (New-Object UAReadArguments($endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10845")), (New-Object UAReadArguments($endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10853")), (New-Object UAReadArguments($endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10855")) )) foreach ($valueResult in $valueResultArray) { if ($valueResult.Succeeded) { Write-Host "Value: $($valueResult.Value)" } else { Write-Host "*** Failure: $($valueResult.ErrorMessageBrief)" } } # Example output: # #Value: 8 #Value: -8.06803E+21 #Value: Strawberry Pig Banana Snake Mango Purple Grape Monkey Purple? Blueberry Lemon^
' This example shows how to read the Value attributes of 3 different nodes at once. Using the same method, it is also possible ' to read multiple attributes of the same node. Imports System Imports OpcLabs.BaseLib.OperationModel Imports OpcLabs.EasyOpc.UA Imports OpcLabs.EasyOpc.UA.OperationModel Namespace _EasyUAClient Partial Friend Class ReadMultipleValues Public Shared Sub Main1() ' Define which server we will work with. Dim endpointDescriptor As UAEndpointDescriptor = "opc.tcp://opcua.demo-this.com:51210/UA/SampleServer" ' or "http://opcua.demo-this.com:51211/UA/SampleServer" (currently not supported) ' or "https://opcua.demo-this.com:51212/UA/SampleServer/" ' Instantiate the client object Dim client = New EasyUAClient() ' Obtain values. By default, the Value attributes of the nodes will be read. Dim valueResultArray() As ValueResult = client.ReadMultipleValues(New UAReadArguments() _ { New UAReadArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10845"), New UAReadArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10853"), New UAReadArguments(endpointDescriptor, "nsu=http://test.org/UA/Data/ ;i=10855") } ) ' Display results For Each valueResult As ValueResult In valueResultArray If valueResult.Succeeded Then Console.WriteLine("Value: {0}", valueResult.Value) Else Console.WriteLine("*** Failure: {0}", valueResult.ErrorMessageBrief) End If Next valueResult ' Example output: ' 'Value: 8 'Value: -8.06803E+21 'Value: Strawberry Pig Banana Snake Mango Purple Grape Monkey Purple? Blueberry Lemon^ End Sub End Class End Namespace
System.Object
OpcLabs.BaseLib.Object2
OpcLabs.BaseLib.Info
OpcLabs.BaseLib.OperationModel.OperationResult
OpcLabs.BaseLib.OperationModel.ValueResult
OpcLabs.BaseLib.OperationModel.Generic.ValueResult<T>